ASP.NET MVC Interview Questions and Answers
Q1. What is MVC
Ans: Model–view–controller (MVC) is a software design pattern for implementing user interfaces on computers. It divides a given software application into three interconnected parts, so as to separate internal representations of information from the ways that information is presented to or accepted from the user.
Q2. As an ASP.NET MVC is a framework for building web applications using a MVC (Model View Controller) ?
Ans: A Model , which represents the underlying, logical structure of data in a software application and the high-level class associated with it. This object model does not contain any information about the user interface.
- A View , which is a collection of classes representing the elements in the user interface (all of the things the user can see and respond to on the screen, such as buttons, display boxes, and so forth)
- A Controller , which represents the classes connecting the model and the view, and is used to communicate between classes in the model and view. The MVC model also provides full control over HTML, CSS, and JavaScript.
Q3. Can you explain the complete flow of MVC?
Ans: Below are the steps how control flows in MVC (Model, view and controller) architecture:-
1.All end user requests are first sent to the controller.
2.The controller depending on the request decides which model to load. The controller loads the model and attachesthe model with the appropriate view.
3.The final view is then attached with the model data and sent as a response to the end user on the browser
Q4. What are the different types of action results in MVC?
Ans: In MVC there are 12 results. Among them ActionResult class is a base class for all action results. The 11 sub classes are given bellow:
- ViewResult– renders a view as a web page to the response stream
- PartialViewResult– renders a view inside another view
- EmptyResult– returns a empty or null result
- RedirectResult– redirect to another action method (HTTP redirection)
- RedirectToRouteResult– redirect to another action method (HTTP redirection) that is determined by the routing engine, based on a given route
- JsonResult– returns a JSON object form a ViewData
- JavaScriptResult– returns a script code that can be executed on the client
- ContentResult– returns a user-defined content type
- FileContentResult– returns a file to the client
- FileStreamResult– returns a file to the client, which is supplied by a Stream
- FilePathResult– returns a file to the client
Q5. What are routs in MVC and where to configure it?
Ans: Routing is a way to process the incoming url that is more descriptive and give desired response. A route is a URL pattern that is mapped to a handler. The handler can be a physical file, such as a .aspx file in a Web Forms application. A handler can also be a class that processes the request, such as a controller in an MVC application. To define a route, you create an instance of the Route class by specifying the URL pattern, the handler, and optionally a name for the route.
Q6. Explain the difference between 3-layer architecture and MVC architecture.
Ans: MVC is an evolution of a three layered traditional architecture. Many components of the three layered architecture are the part of MVC.
A fundamental rule in three tier architecture is the client tier never communicate directly with the data tier. In a three-tier model all communication must pass through the middle tier.
MVC architecture is triangular. The view sends updates to the controller, the controller updates the model, and the view gets updated directly from the model.
Q7. What Are Action Filters in MVC?
Ans: Action Filters: Action filters are used to implement logic that gets executed before and after a controller action executes.
Q8. Is MVC suitable for both windows,and web application?
Ans: MVC architecture is suited for web application than windows. For window application MVP i.e. “Model view presenter”is more applicable.IfyouareusingWPFandSLMVVMismoresuitableduetobindings
Q9. What is Razor Engine and how it works?
Ans: Razor ia an ASP MVC view engine that lets you to write server-based code in your view to create a dynamic content the render it into availd HTML
Q10.How to implement Windows authentication in MVC?
Ans: For Windows authentication, at first we need to change the web.config file and set the authentication mode to Windows. A sample code for that is given bellow:
<authentication mode="Windows"/>
<authorization>
<deny users="?"/>
</authorization>
Then in the controller or on the action, we can use the Authorize attribute which specifies which users have access to these controllers and actions. A sample code for that is given bellow, where only the user “Administrator” can access it.
[Authorize(Users= @”WIN-3LI600MWLQN\Administrator”)]
public class StartController : Controller
{
//
// GET: /Start/
[Authorize(Users = @"WIN-3LI600MWLQN\Administrator")]
public ActionResult Index()
{
return View("MyView");
}
}
Q11. Mention three ways to pass data between the controllers and views, and when to use each of them?
Ans: ViewData: It is available for the current request only and requires typecasting for complex data type.
ViewBag: Dynamic property that takes advantage of the new dynamic features in C# 4.0, also available for the current request only. If redirection occurs, then its value becomes null and doesn’t require typecasting for complex data type.
TempData: used to pass data from the current request to the next request, keeps the information for the time of an HTTP Request. This means only from one page to another.
Q12. Explain the MVC application life cycle.
Ans: Any web application first understands the request and depending on type of request, it sends out appropriate response. MVC application life cycle is not different, it has two main phases, first creating the request object and second sending our response to the browser.
The request object creation has four major steps,
Step 1: Fill route
Every MVC request is mapped to route table which specifies which controller and action to be invoked. If it is the first request, it fills the route table with route collection. This filling of route table happens in the global.asaxfile.
Step 2: Fetch route
Depending on the URL sent "UrlRoutingModule" searches the route table to create "RouteData" object. RouteData has the details of which controller and action to invoke.
Step 3: Request context created
The "RouteData" object is used to create the "RequestContext" object.
Step 4: Controller instance created
The coming request object is sent to "MvcHandler" instance to create the object of controller class. Once the controller class object is created, it calls the "Execute" method of the controller class.
Creating Response object:
This phase has two steps - Executing the action and finally sending the response as a result to the view.
Q13. What are HTML Helpers and why to use it?
Ans: HtmlHelper class generates html elements using the model class object in razor view. It binds the model object to html elements to display value of model properties into html elements and also assigns the value of the html elements to the model properties while submitting web form. So always use HtmlHelper class in razor view instead of writing html tags manually.
Q14. What do you know about bundling and minification in MVC?
Ans: In MVC, bundling and minification helps us improve request load times of a page. It increases the performance of the applications.
Q15. What is RESTfull APIs?
Ans: REST stands for REpresentational State Transfer. REST is web standards based architecture and uses HTTP Protocol for data communication. It revolves around resource where every component is a resource and a resource is accessed by a common interface using HTTP standard methods
In REST architecture, a REST Server simply provides access to resources and REST client accesses and presents the resources. Here each resource is identified by URIs/ global IDs. REST uses various representations to represent a resource like text, JSON and XML. Now a days JSON is the most popular format being used in web services.
Q16. What is the latest version of MVC?
Ans: When this note was written, four versions where released of MVC. MVC 1 , MVC 2, MVC 3 and MVC 4. So the latest isMVC 4
Q17. What is Output Caching in MVC?
Ans: It enables us to cache the content returned by any controller method so that the same content does not need to be generated each time the same controller method is invoked. Output Caching has huge advantages, such as it reduces server round trips, reduces database server round trips, reduces network traffic etc. The main purpose of using Output Caching is to improve the performance of the application.
Q18. What is the difference between each version of MVC?
Ans: Below is a detail table of differences. But during interviewit’s difficult to talk about all of them due to timelimitation.So I have highlighted important differences which you can run through before the interviewer.
MVC 2
MVC 3
MVC 4
Client-Side Validation TemplatedHelpers Areas AsynchronousControllers
Html.ValidationSummaryHelper Method DefaultValueAttribute inActionMethod Parameters Binding BinaryData with Model Binders DataAnnotationsAttributes Model-Validator Providers NewRequireHttpsAttribute Action FilterTemplated Helpers Display Model-LevelErrors
Razor
Readymade project templates
HTML 5 enabled templatesSupportfor Multiple View EnginesJavaScriptand Ajax
Model Validation Improvements
ASP.NET Web API
Refreshed and modernized defaultproject templatesNew mobile projecttemplate
Many new features to support mobileapps
Enhanced support for asynchronousmethods
Q19. What is Bundling and Minification in MVC?
Ans: Bundling and minification are two techniques you can use to improve request load time.
Bundling helps you to download files of same type using one request instead of multiple requests. This way you can download styles and scripts using less requests than it takes to request all files separately.
Minification performs a variety of different code optimizations to scripts or css, such as removing unnecessary white space,comments and shortening variable names to one character.
Q20. What is minification and how to implement minification in MVC?
Ans: Minification is a process to remove the blank space, comments etc.
The implementation of minification is as like as bundling. That means when we implement bundling minification is also implemented automatically. A sample JavaScript codes with comments:
// This is test comments
var x = 5;
x = x + 2;
x = x * 3;
After implementing minification the JavaScript code looks like:
var x=5;x=x+12x=x*3
Q21. What is Partial View in MVC?
Ans: A partial view is a component built by the developer to re-use a bulk of HTML that can be inserted into an existing DOM. Most commonly, partial views are used to componentize Razor views and make them easier to build and update. Partial views can also be returned directly from controller methods.
Q22. What about static files in ASP.NET Core (MVC)?
Ans: All static files are now (by default) located inside of wwwrootfolder. You store your CSS, JS, images, fonts and others static content inside of it.
You May Also Like
These Related Stories
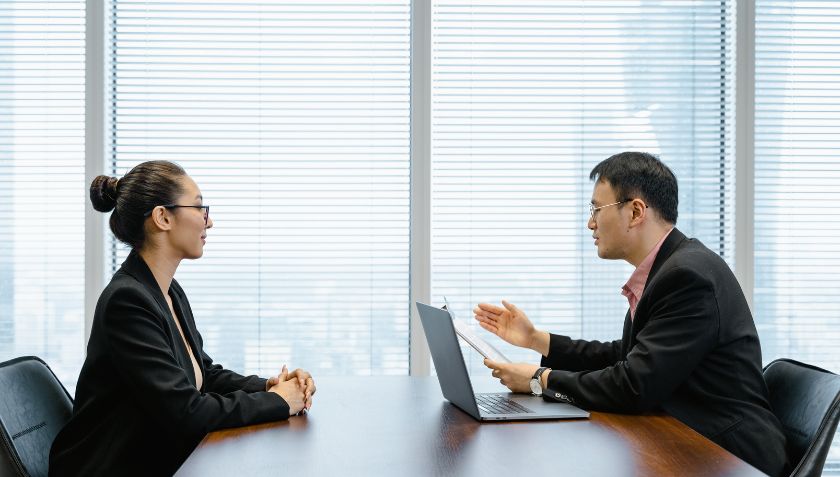
ASP.NET Interview Questions and Answers For Experienced
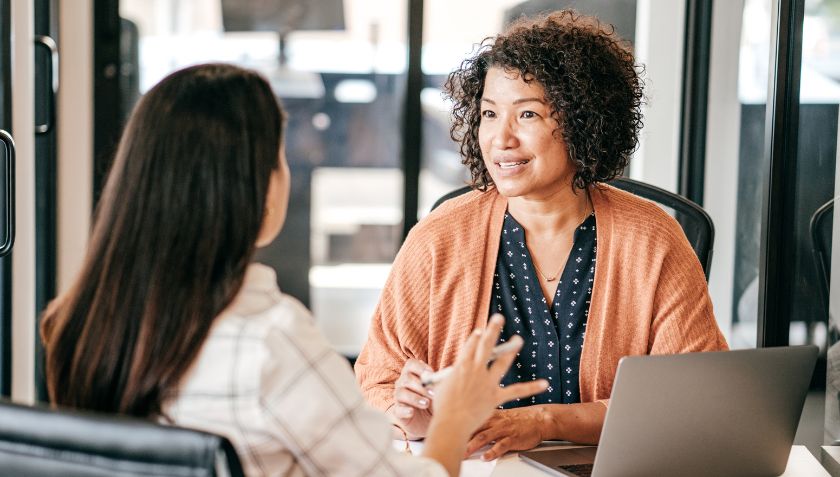
ASP .Net Web API Essentials using C# Interview Questions
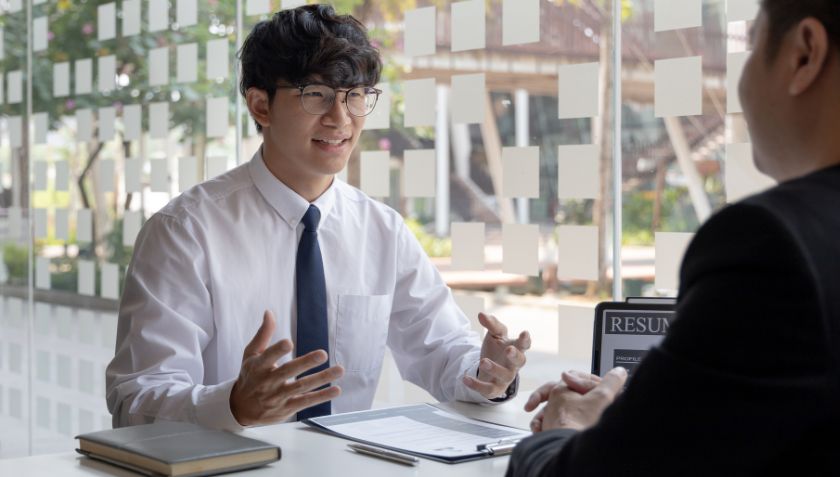
No Comments Yet
Let us know what you think