ASP.NET Interview Questions and Answers For Experienced
Q1. What is ASP.NET?
Ans: ASP.NET was evolved in direct acknowledgment to the problems that developers had with standard ASP. Since ASP is in such wide use, Microsoft guaranteed that ASP scripts execute without modification on a machine with the .NET Framework (the ASP engine, ASP.DLL, is not modified when installing the .NET Framework). Thus, IIS can house both ASP and ASP.NET scripts on the same machine.
Q2. What is XHTML? Are ASP.NET Pages compliant with XHTML?
Ans: In plain words, XHTML is a stricter and cleaner version of HTML. XHTML stands for EXtensible Hypertext Markup Language and is a W3C Recommendation.
Yes, ASP.NET 2.0 Pages are XHTML compliant. However the facility has been given to the user to add the applicable document type declaration.
Q3. Does ViewState affect performance? What is the ideal size of a ViewState? How can you compress a viewstate?
Ans: Viewstate stores the state of controls in HTML hidden fields. At times, this information can grow in capacity. This does affect the comprehensive responsiveness of the page, thereby affecting performance. The optimal size of a viewstate should be not more than 25-30% of the page size.
ViewState can be compressed to almost 50% of its size. .NET also provides the GZipStream orDeflateStream to compress viewstate
Q4. How can you detect if a viewstate has been tampered?
Ans: By setting the EnableViewStateMac to true in the @Page directive. This attribute checks the encoded and encrypted viewstate for tampering.
Q5. Can the App_Code folder contain source code files in different programming languages?
Ans: No. All source code files stored in the root App_Code folder must be in the same programming language. However, you can create two subfolders inside the App_Code and then add both C# and VB.NET in the respective subfolders. You also have to add configuration settings in the web.config for this to work.
Q6. Can I deploy the application without deploying the source code on the server?
Ans: Yes. You can complicate your code by using a new precompilation process called ‘precompilation for deployment’. You can use the aspnet_compiler.exe to precompile a site. This process builds each page in your web application into a single application DLL and some placeholder files. These files can then be deployed to the server.
You can also conclude the same task using Visual Studio 2005 by using the Build->Publish menu.
Q7. Can I use different programming languages in the same application?
Ans: Yes. Each page can be written with a different programming language in the same application. You can create a few pages in C# and a few in VB.NET.
Q8. How to secure your connection string information?
Ans: By using the Protected Configuration feature.
Q9. What is Cross Page Posting? How is it done?
Ans: By default, ASP.NET submits a form to the same page. In cross-page posting, the form is submitted to a different page. This is done by setting the ‘PostBackUrl’ property of the button(that causes postback) to the desired page. In the code-behind of the page to which the form has been posted, use the ‘FindControl’method of the ‘PreviousPage’ property to reference the data of the control in the first page.
Q10. Can you change a Master Page dynamically at runtime? How?
Ans: Yes. To change a master page, set the MasterPageFile property to point to the .master page during the PreInit page event.
Q11. How to secure your configuration files to be accessed remotely by unauthorized users?
Ans: ASP.NET configures IIS to deny access to any user that requests access to the Machine.config or Web.config files.
Q12. How to configure ASP.NET applications that are running on a remote machine?
Ans: You can use the Web Site Administration Tool to configure remote websites.
Q13. How many web.config files can I have in an application?
Ans: You can keep multiple web.config files in an application. You can place a Web.config file inside a folder or wherever you need (apart from some exceptions) to override the configuration settings that are inherited from a configuration file located at a higher level in the hierarchy.
Q14. What is the difference between Response.Write and Response.Output.Write?
Ans: As quoted by Scott Hanselman, the short answer is that the latter gives you String.Format-style output and the former doesn't.
Q15. How do you apply Themes to an entire application?
Ans: By specifying the theme in the web.config file.
< configuration > < system.web > < pages theme=”BlueMoon” /> </ system.web > </ configuration > |
Q16. How do you exclude an ASP.NET page from using Themes?
Ans: To remove themes from your page, use the EnableTheming attribute of the Page directive.
Q17. Your client complains that he has a large form that collects user input. He wants to break the form into sections, keeping the information in the forms related. Which control will you use?
Ans: The ASP.NET Wizard Control.
Q18. webservices support data reader?
Ans: No. However it does support a dataset.
Q19. Can you programmatically access IIS configuration settings?
Ans: Yes. You can use ADSI, WMI, or COM interfaces to configure IIS programmatically.
Q20. What is caching in ASP.NET?
Ans: Caching is one of the most interesting concept and operation in ASP.NET. If you can handle it, you can run any web application by applying the caching concept depending on the requirements.Caching is for providing solutions or the results to the users depending on their request, admin needs to recreate the pages often depending on user requests…STOP!!! "A cache simply stores the output generated by a page in the memory and this saved output (cache) will serve us (users) in the future.
Types
- Page Caching
- Fragment Caching
- Data Caching
Q21. What are the major events in global.aspx?
Ans: The Global.asax file, which is derived from the HttpApplication class, maintains a pool of HttpApplication objects, and assigns them to applications as needed. The Global.asax file contains the following events:
- Application_Init
- Application_Disposed
- Application_Error
- Application_Start
- Application_End
- Application_BeginReques
Q22. What is use of the AutoEventWireup attribute in the Page directive ?
Ans: The AutoEventWireUp is a boolean attribute that allows automatic wireup of page events when this attribute is set to true on the page. It is set to True by default for a C# web form whereas it is set as False for VB.NET forms. Pages developed with Visual Studio .NET have this attribute set to false, and page events are individually tied to handlers.
Q23. What happens when you change the web.config file at run time?
Ans: ASP.NET invalidates the existing cache and assembles a new cache. Then ASP.NET automatically restarts the application to apply the changes.
Q24. What are Cookies in ASP.NET?
Ans: Cookies are a State Management Technique that can store the values of control after a post-back. Cookies can store user-specific Information on the client's machine like when the user last visited your site. Cookies are also known by many names, such as HTTP Cookies, Browser Cookies, Web Cookies, Session Cookies and so on. Basically cookies are a small text file sent by the web server and saved by the Web Browser on the client's machine.
Q25. What is the code behind and Inline Code?Code Behind
Ans: Code Behind refers to the code for an ASP.NET Web page that is written in a separate class file that can have the extension of .aspx.cs or .aspx.vb depending on the language used. Here the code is compiled into a separate class from which the .aspx file derives. You can write the code in a separate .cs or .vb code file for each .aspx page. One major point of Code Behind is that the code for all the Web pages is compiled into a DLL file that allows the web pages to be hosted free from any Inline Server Code.
Inline Code
Inline Code refers to the code that is written inside an ASP.NET Web Page that has an extension of .aspx. It allows the code to be written along with the HTML source code using a <Script> tag. It's major point is that since it's physically in the .aspx file it's deployed with the Web Form page whenever the Web Page is deployed.
Q26. What is the ASP.NET page life Cycle?
Ans: When a page is requested by the user from the browser, the request goes through a series of steps and many things happen in the background to produce the output or send the response back to the client. The periods between the request and response of a page is called the "Page Life Cycle".
- Request: Start of the life cycle (sent by the user).
- Response: End of the life cycle (sent by the server).
There are four stages that occur during the Page Life Cycle before the HTML Response is returned to the client. Later in this article we"ll study all these stages and their sub events.
- Initialization :During this stage the IsPostback property is set. The page determines whether the request is a Postback (old request) or if this is the first time the page is being processed (new request). Controls on the page are available and each control's UniqueID property is set. Now if the current request is a postback then the data has not been loaded and the value of the controls have not yet been restored from the view state.
- Loading :At this stage if the request is a Postback then it loads the data from the view state.
- Rendering : Before rendering, the View State is saved for the page and its controls. During this phase, the page calls the render method for each control, providing a text writer that writes its output to the OutputStream of the page's Response property.
- Unloading :Unload is called after the page has been fully rendered, sent to the client and is ready to be discarded. At this point also the page properties such as Response and Request are unloaded.
Q27. What is the Difference between session and caching?
Ans: The first main difference between session and caching is: a session is per-user based but caching is not per-user based, So what does that mean? Session data is stored at the user level but caching data is stored at the application level and shared by all the users. It means that it is simply session data that will be different for the various users for all the various users, session memory will be allocated differently on the server but for the caching only one memory will be allocated on the server and if one user modifies the data of the cache for all, the user data will be modified.
Q28. Explain Web Services in ASP.NET?
Ans: A Web Service is a software program that uses XML to exchange information with other software via common internet protocols. In a simple sense, Web Services are a way for interacting with objects over the Internet.
A web service is:
- Language Independent.
- Protocol Independent.
- Platform Independent.
- It assumes a stateless service architecture.
- Scalable (e.g. multiplying two numbers together to an entire customer-relationship management system).
- Programmable (encapsulates a task).
- Based on XML (open, text-based standard).
- Self-describing (metadata for access and use).
- Discoverable (search and locate in registries)- ability of applications and developers to search for and locate desired Web services through registries. This is based on UDDI.
Q29. Explain the concepts of Globalization and Localization in .NET?
Ans: Localization means "process of translating resources for a specific culture", and Globalization means "process of designing applications that can adapt to different cultures".
- Proper Globalization: Your application should be able to Accept, Verify, and Display all global kind of data. It should well also be able to operate over this data, accordingly. We will discuss more about this "Accordingly operations over diff. culture data".
- Localizability and Localization: Localizability stands for clearly separating the components of culture based operations regarding the user interface, and other operations from the executable code.
.NET framework has greatly simplified the task of creating the applications targeting the clients of multiple cultures. The namespaces involved in creation of globalize, localizing applications are:
- System.Globalization
- System.Resources
- System.Text
Q30. What is tracing in .NET?
Ans: Tracing helps to see the information of issues at the runtime of the application. By default Tracing is disabled.
Tracing has the following important features:
- We can see the execution path of the page and application using the debug statement.
- We can access and manipulate trace messages programmatically.
- We can see the most recent tracing of the data.
Tracing can be done with the following 2 types.
- Page Level: When the trace output is displayed on the page and for the page-level tracing we need to set the property of tracing at the page level.<%@ Page Trace="true" Language="C#"
- Application: Level: In Application-Level tracing the information is stored for each request of the application. The default number of requests to store is 10. But if you want to increase the number of requests and discard the older request and display a recent request then you need to set the property in the web.config file.<trace enabled="true"/>
You May Also Like
These Related Stories
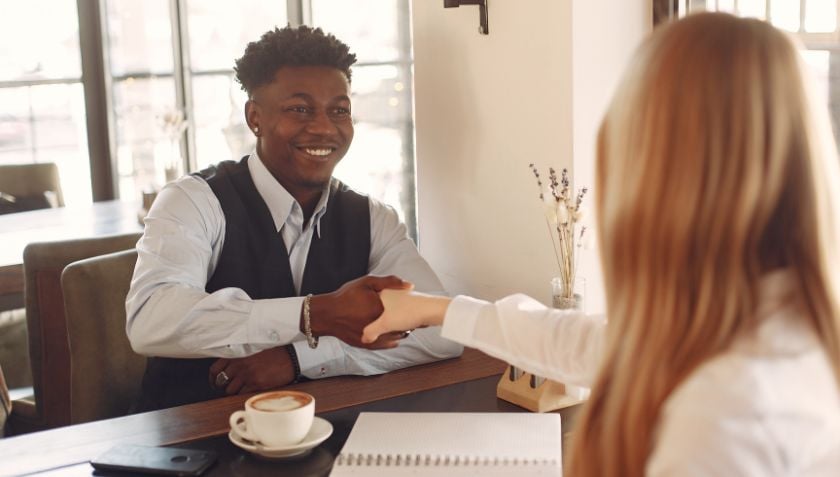
ASP.NET MVC Interview Questions and Answers
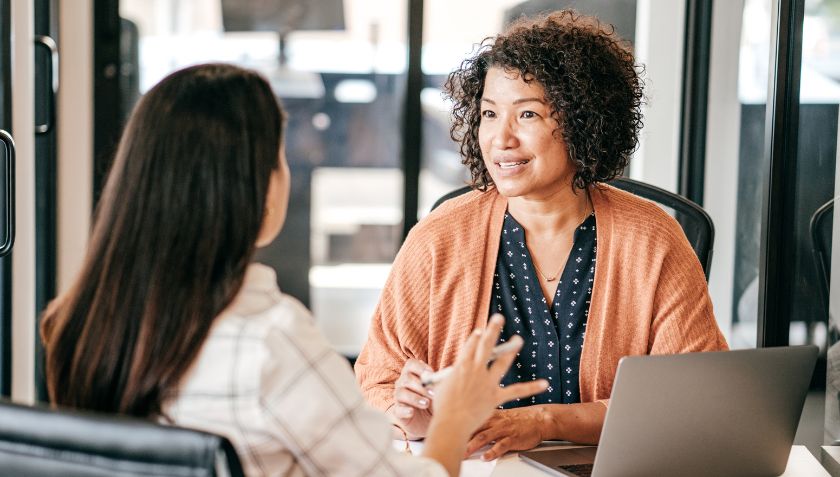
ASP .Net Web API Essentials using C# Interview Questions
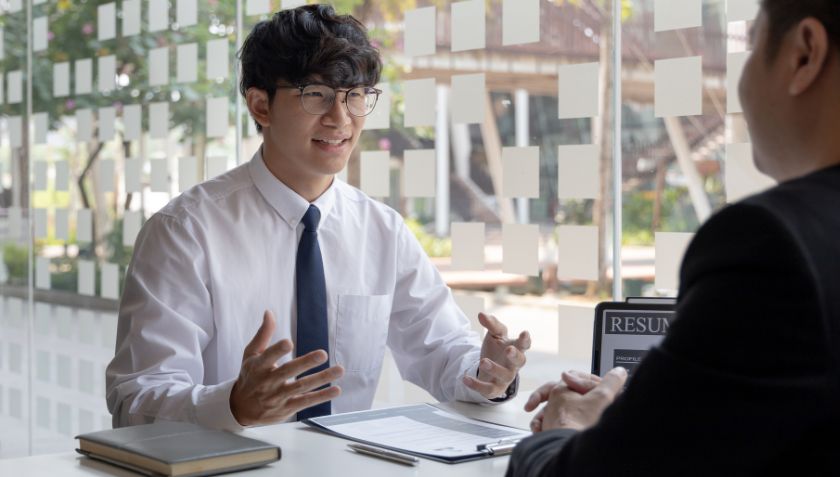
No Comments Yet
Let us know what you think