Spring MVC Interview Questions and Answers For Experienced
.jpg?width=840&name=Interview%20Questions%20(13).jpg)
Q1. What is "Spring Framework" ?
Ans: With the two core concepts of Aspect-Oriented and Dependency Injection, Spring is one of the most popular and widely used Java EE frameworks. Through dependency injection it can provide tight coupling between various components. The cross-cutting tasks such as authentication and logging can be provided through Spring framework and the aspect-oriented programming can be implemented through this as well.
Spring framework is a featured framework which can provide several modules and lots of features for the specific tasks such as Spring JDBC and Spring MVC. Working with Spring is quite easy and a fun activity due to the presence of a number of online communities and resources.
Q2. Why Spring Framework is needed?
Ans: Spring framework is needed because it is –
- Very Light Weight Container
- Framework
- IOC
- AOP
Q3. What are the advantages of Spring Framework?
Ans: Spring framework offers a number of advantages which includes the following:
- The direct dependency among several components can be reduced through Spring IoC containers. They are responsible to create the beans, which are injected between the components and to reduce the dependency.
- As the business logic does not have any direct dependency on the implementation class of actual resource so it becomes quite easier to write the Unit test cases. The test configurations can be easily written and the configurations can be injected easily for testing purposes.
- The amount of boilerplate code is also reduced e,g. to open or close the resource or to initialize an JdbcTemplate class can also remove the boilerplate code required in JDBC programming.
- Spring framework can help us in keeping the application lightweight say e.g if Spring transaction management feature is not required, then the user needs not to add that dependency to the project.
- Spring framework can support a number of Java EE framework features even more than Spring is always top of the technologies like to write better naïve android application user can use Spring project for Android. So it can be a complete package and user need not have any separate package for the work.
Q4. Name some popular and mostly used Spring Modules.
Ans: Some important and mostly used Spring modules are:
- Spring Context- Used for Dependency Injection
- Spring AOP- Used to implement aspect-oriented programming
- Spring DAO- Used for database operations with the help of DAO patterns
- Spring JDBC- uSed for DataSource and JDBC support
- Spring ORM- Used for creating web applications
- Spring Web Module- Used to create the web applications
- Spring MVC- It is also known as Model-View-Controller used to create and implement the web applications
Q5. What is Spring Bean?
Ans: Any class of Spring framework, which is initialized by Spring IoC container is known as Spring Bean. We can get the Spring Bean instance through Spring Application Context. The life-cycle of Spring Bean can be managed by Spring IoC container.
Q6. Explain Aspect Oriented Programming.
Ans: Some cross-cutting concerns for any enterprise level application like transaction management, data validation, authentication and logging in for various application modules and objects are most valuable. In case of object-oriented programming such application modularity is implemented by classes while in AOP it is achieved by Aspects.
Q7. What are the methods of Bean Life Cycle?
Ans: There are two important methods of Bean life cycle:
- Setup – called when bean is loaded into container
- Teardown – called when bean is unloaded into container
Q8. What are the features of Spring Framework?
Ans: Spring framework is based on two main concepts known as aspect-oriented programming and dependency injection. Few features of Spring framework are:
- Spring framework is lightweight and with little overhead for the developers
- The container manager of Spring framework can manage Spring Bean life cycle and any project specific configurations like JNDI lookup.
- It can support JDBC operations, transaction management, exception handling and file uploading like little configurations either by using annotations or with the help of Spring bean configuration file.
- This framework can be used to develop the web applications and web services both which can return JSON and XML responses
- Through inversion of control and dependency injection concepts you can develop the independent components easily. Through spring containers these components can be wired easily.
Q9. What is dependency injection?
Ans: Dependency injection is a concept which is implemented through the design patterns. It can remove the hard-coded dependency and can make the application loosely coupled, maintainable and extendable. The dependency resolution can also be moved from compile time to run time through these design patterns. It provides following benefits:
- Boilerplate Code Reduction
- Separation of Concerns
- Easy Unit Testing
- ConfigurableComponents
Google Guice can also be used for dependency injection and the processes can be automated through this. If we want to implement any additional concept along with dependency injection then Spring is the best choice for that.
Q10. What is the front controller class of Spring MVC?
Ans: A front controller is defined as “a controller which handles all requests for a Web Application.” DispatcherServlet (actually a servlet) is the front controller in Spring MVC that intercepts every request and then dispatches/forwards requests to an appropriate controller.
When a web request is sent to a Spring MVC application, dispatcher servlet first receives the request. Then it organizes the different components configured in Spring’s web application context (e.g. actual request handler controller and view resolvers) or annotations present in the controller itself, all needed to handle the request.
Q11. What is Spring MVC Interceptor and how to use it?
Ans: As you know about servlet filters that they can pre-handle and post-handle every web request they serve — before and after it’s handled by that servlet. In the similar way, you can use HandlerInterceptor interface in your spring mvc application to pre-handle and post-handle web requests that are handled by Spring MVC controllers. These handlers are mostly used to manipulate the model attributes returned/submitted they are passed to the views/controllers.
A handler interceptor can be registered for particular URL mappings, so it only intercepts requests mapped to certain URLs. Each handler interceptor must implement the HandlerInterceptor interface, which contains three callback methods for you to implement: preHandle(), postHandle() and afterCompletion().
Problem with HandlerInterceptor interface is that your new class will have to implement all three methods irrespective of whether it is needed or not. To avoid overriding, you can use HandlerInterceptorAdapter class. This class implementsHandlerInterceptor and provide default blank implementations.
Q12. How does Spring MVC provide validation support?
Ans: Spring supports validations primarily into two ways.
Using JSR-303 Annotations and any reference implementation e.g. Hibernate Validator
- Using custom implementation of org.springframework.validation.Validator interface
Q13. Are Singleton beans thread safe in Spring Framework?
Ans: No, singleton beans are not thread-safe in Spring framework.
Q14. What is the difference between concern and cross-cutting concern in Spring AOP?
Ans:
Concern − Concern is behavior which we want to have in a module of an application. Concern may be defined as a functionality we want to implement. Issues in which we are interested define our concerns.
Cross-cutting concern − It's a concern which is applicable throughout the application and it affects the entire application. e.g. logging , security and data transfer are the concerns which are needed in almost every module of an application, hence are cross-cutting concerns.
Q15. How to use Tomcat JNDI DataSource in Spring Web Application?
Ans: For using servlet container configured JNDI DataSource, we need to configure it in the spring bean configuration file and then inject it to spring beans as dependencies. Then we can use it with JdbcTemplate to perform database operations.
< bean id="dataSource" class="org.springframework.jndi.JndiObjectFactoryBean">
< property name="jndiName" value="java:comp/env/jdbc/MySQLDB"/>
< /bean>
Q16. What is Bean Factory?
Ans: Bean Factory is core of the spring framework and, it is a Lightweight container which loads bean definitions and manages your beans. Beans are configured using XML file and manage singleton defined bean. It is also responsible for life cycle methods and injects dependencies. It also removes adhoc singletons and factories.
Q17. What are the different types of Events of Listeners?
Ans: Following are the different types of events of listeners:
- ContextClosedEvent – This event is called when the context is closed.
- ContextRefreshedEvent – This event is called when context is initialized or refreshed
- RequestHandledEvent – This event is called when the web context handles request
Q18. What is the difference between Bean Factory and ApplicationContext?
Ans:
- Application contexts provide a means for resolving text messages, including support for i18n of those messages.
- Application contexts provide a generic way to load file resources, such as images.
- Application contexts can publish events to beans that are registered as listeners.
- Certain operations on the container or beans in the container, which have to be handled in a programmatic fashion with a bean factory, can be handled declaratively in an application context.
- The application context implements MessageSource, an interface used to obtain localized messages, with the actual implementation being pluggable.
Q19. What is Controller in Spring MVC framework?
Ans: Controllers provide access to the application behavior that you typically define through a service interface. Controllers interpret user input and transform it into a model that is represented to the user by the view. Spring implements a controller in a very abstract way, which enables you to create a wide variety of controllers.
Q20. What are the ways to access Hibernate by using Spring?
Ans: There are two ways to access hibernate using spring −
- Inversion of Control with a Hibernate Template and Callback.
- Extending HibernateDAOSupport and Applying an AOP Interceptor node.
You May Also Like
These Related Stories
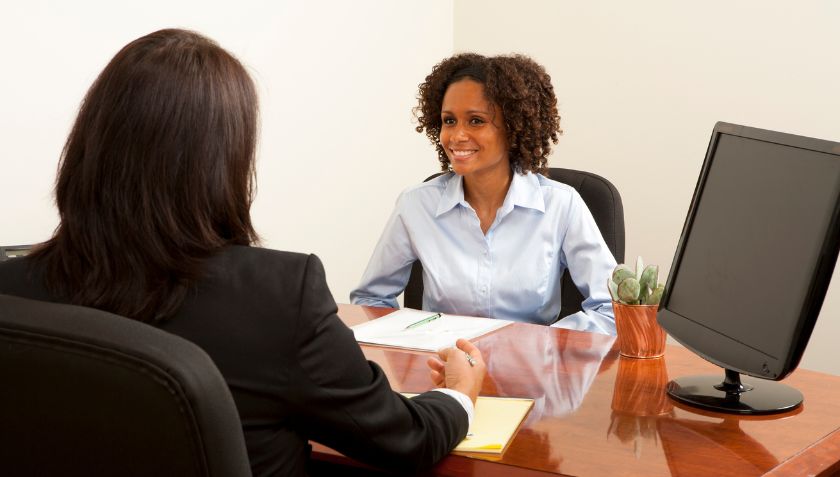
Spring Interview Questions and Answers
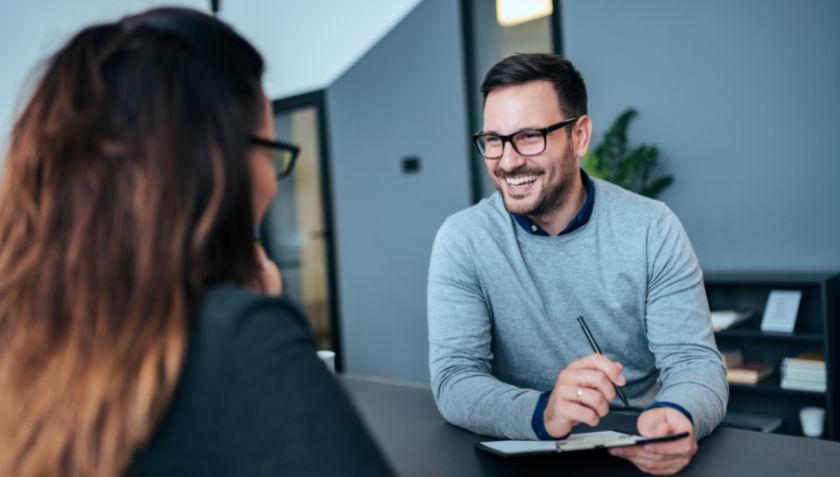
Spring Interview Questions and Answers for Experienced
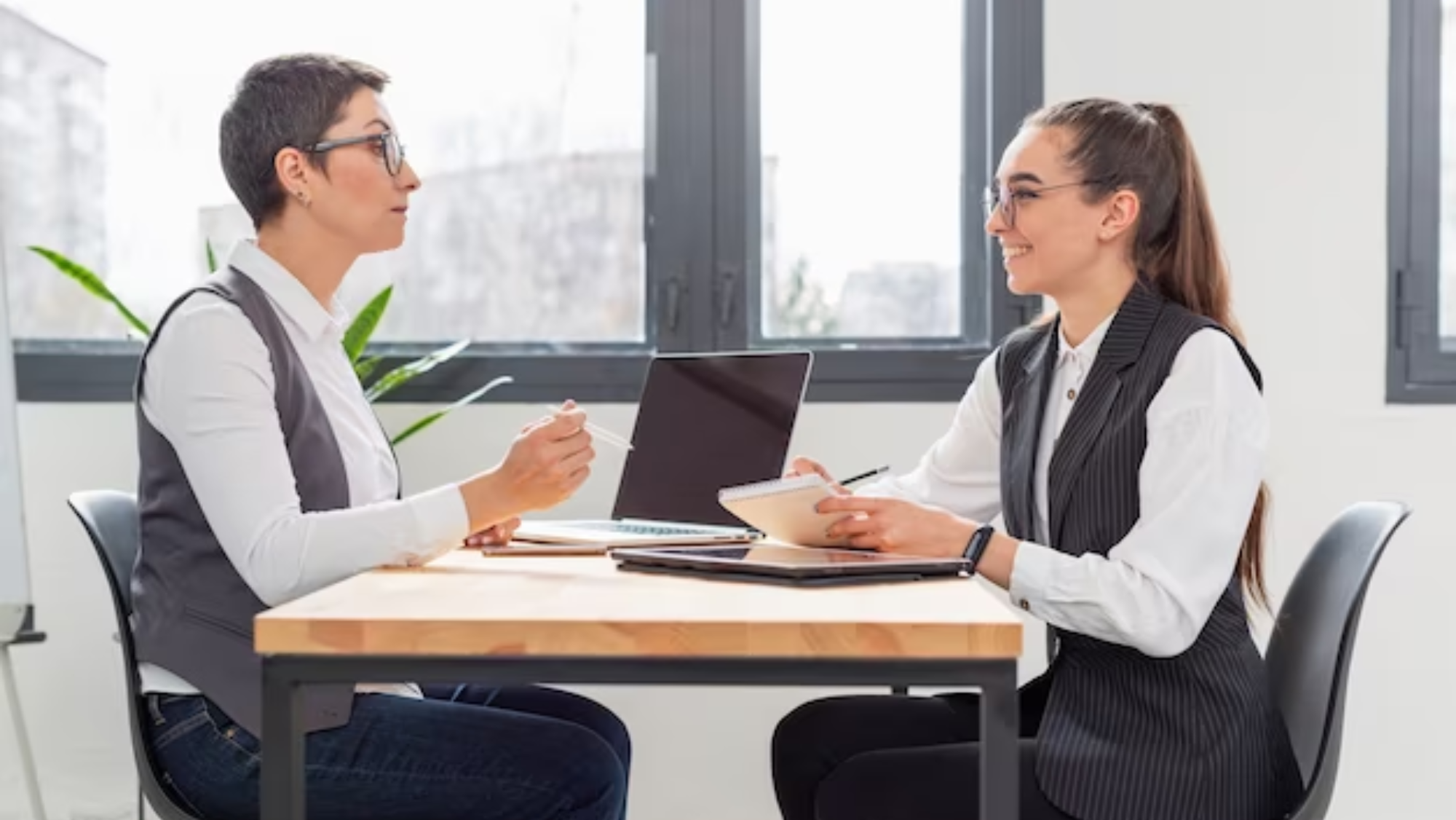
No Comments Yet
Let us know what you think