PHP Interview Questions and Answers for Freshers 2018
Q1. What is PHP?
PHP is server side scripting language. It is the most widely used web technology to create dynamic web pages. There are many PHP based frameworks and Open sources available for free to use. Some examples include WordPress, Drupal, Laravel etc. We can embed PHP with HTML and can further write server-side code with PHP for web development.
Q2. Which is the latest version of PHP?
Stable release: 7.1.4 / 13 Apr 2017; http://php.net/downloads.php
Q3. What are the extensions of PHP File?
.php, .phtml, .php3, .php4, .php5, .php7, .phps .
Q4. What is the difference between include(), include_once() and require_once()
The include() statement includes and evaluates a specified line i.e. it will include a file based in the given path. require() does the same thing expect upon failure it will generate a fatal error and halt the script whereas include() will just gives a warning and allow script to continue. require_once() will check if the file already has been included and if so it will not include the file again.
Q5. Differences between GET, POST and REQUEST methods ?
GET and POST are used to send information from client browser to web server. In case of GET the information is send via GET method in name/value pair and is URL encoded. The default GET has a limit of 512 characters. The POST method transfers the information via HTTP Headers. The POST method does not have any restriction in data size to be sent. POST is used for sending data securely and ASCII and binary type’s data. The $_REQUEST contains the content of both $_GET, $_POST and $_COOKIE.
Q6. Explain how PHP sends output to Browser? OR How a file is processed when it sends output to browser ?
1. Open website http://www.tutorialmines.net
2. Request send to server of http://www.tutorialmines.net
3. Call PHP Files.
4. PHP Scripts are loaded into memory and compiled into Zend opcode.
5. These opcodes are executed and the HTML generated.
6. Same HTML is send back to Browser.
Q7. What are the different errors in PHP?
There are 4 basically types of error.
Parse Error – Commonly caused due to syntax mistakes in codes e.g. missing semicolon, mismatch brackets.
Fatal Error – These are basically run time errors which are caused when you try to access what can’t be done. E.g. accessing a dead object, or trying to use a function that hasn’t been declared.
Warning Error – These occurs when u try to include a file that is not present, or delete a file that is not on the server. This will not halt the script; it will give the notice and continue with the next line of the script.
Notice Error – These errors occurs when u try to use a variable that hasn’t been declared, this will not halt the script, It will give the notice and continue with the next line of the script.
Q8. What are the various methods/ways to pass data from one web page to another web page?
The different ways to pass the data as follows:
1. Session
2. Cookie
3. Database
4. URL parameters
Q9. What is session and why do we use it?
Session is a super global variable that preserve data across subsequent pages. Session uniquely defines each user with a session ID, so it helps making customized web application where user tracking is needed.
Q10. What is cookie and why do we use it?
Cookie is a small piece of information stored in client browser. It is a technique used to identify a user using the information stored in their browser (if already visited that website) . Using PHP we can both set and get COOKIE.
Q11. What function do we use to find length of string, and length of array?
For finding length of string we use strlen() function and for array we use count() function.
Q12. What are traits in php?
These are PHP mechanisms for code re-usability to ease developers for using code in different class hierarchies.
Q13. List out the predefined classes in PHP?
Directory
stdClass
__PHP_Incomplete_Class
exception
php_user_filter
Q14. How can we change the value of a constant?
We cannot change the value of a constant.
Q15. What is the difference between unset() and unlink() function.
unset() is used to destroy a variable where as unlink() is used to destroy a file.
Q16. How to print current date and time.
Get current date and time
<?php echo date(‘Y-m-d H:i:s’); ?>
|
password_hash
and password_verify
which are respectively used as hashing and checking method. MD5 was used before but now has become obsolete.Q18. What is the difference between explode() and split() functions?
Both are used to split a string to array, the basic difference is that split() uses pattern for splitting and explode()uses a string. explode() is faster than split() as it does not match the string based on regular expression. Also split() is deprecated as of 5.3.0. So using of this function is discouraged.
Q19. What is PDO classes?
The PHP Data Objects (PDO) extension defines a lightweight, consistent interface for accessing databases in PHP. It is a data-access abstraction layer, so no matter what database we use the function to issue queries and fetch data will be same. Using PDO drivers we can connect to database like DB2, Oracle, PostgreSQL etc.
Q20. What are the Formatting and Printing Strings available in PHP?
printf()- Displays a formatted string
sprintf()-Saves a formatted string in a variable
fprintf() -Prints a formatted string to a file
number_format()-Formats numbers as strings
Q21. What is the difference between javascript and PHP?
Javascript is a client side scripting language whereas PHP is a server side scripting language.
Q22. How to get IP address in php?
Simplest way is to use $_SERVER["REMOTE_ADDR"];
but there are many variables like proxies, server, client, system, current and public that may require specific changes.
Q23. What is Constructors and Destructors?
CONSTRUCTOR : PHP allows developers to declare constructor methods for classes. Classes which have a constructor method call this method on each newly-created object, so it is suitable for any initialization that the object may need before it is used.
DESTRUCTORS : PHP 5 introduces a destructor concept similar to that of other object-oriented languages, such as C++. The destructor method will be called as soon as all references to a particular object are removed or when the object is explicitly destroyed or in any order in shutdown sequence
Q24. What is the difference between ID and class in CSS?
The difference between an ID and Class is that an ID can be used to identify one element, whereas a class can be used to identify more than one.
Q25. for image work which library?
We will need to compile PHP with the GD library of image functions for this to work. GD and PHP may also require other libraries, depending on which image formats you want to work with.
Q26. How do we get the current session ID?
<?php
session_start
echo session_id;
?>
Q27. How do we destroy a session.
Destroy a session
PHP:
<?php
session_start;
session_destroy;
?>
Q28. What is an associative array?
Associative arrays are arrays that use named keys that you assign to them.
associative array
Suppose we want to show and hide elements of a div with id div1.
Q30. How can we add change font size using jquery?
Suppose we want change a font size of and div with id div1 from 12px to 18px.
Change font size using jquery
JavaScript:
}
?>
Q34. What are super global variables.
The super global variables as follows:
- $_COOKIE – It Stores all the information in an associative array of variables passed to the current script via HTTP Cookies.
- $_GET – Send data to server which is visible in the URL of the browser. It is not safe to send sensitive using GET method.
- $_POST – Send data to server which is not visible in the URL. When user submits the form with the method set as POST Method.
- $_REQUEST – Its combined array containing values from the $_GET, $_POST and $_COOKIE global variables.
- $_ENV – Get/Set an associative array of variables passed to the current script via the environment method..
- $_FILES – Get an associative array of items uploaded to the current script via the HTTP POST method. Used to upload the file, image and videos etc on server. It contains all the information i.e name, extension, temporary name etc.
- $_SERVER – It saves all information related about headers, paths, and script locations.
- $_SESSION – It contains session variables available to the current script.
- $GLOBALS – PHP super global variable which is used to access global variables from anywhere in the PHP script. Contains all the global variables associated with the current script.
Q35. What are the advantages of PHP mysql?
It is a stable, reliable and powerful solution with advanced features like the following:
- Data Security
- On-Demand Scalability
- High Performance
- Round-the-clock Uptime
- Comprehensive Transactional Support
- Complete Workflow Control
- Reduced Total Cost of Ownership
- The Flexibility of Open Source
You May Also Like
These Related Stories
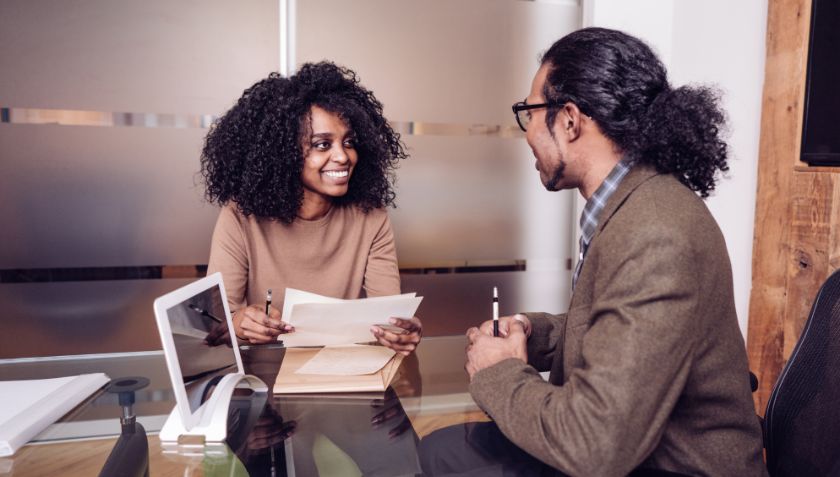
Typo3 CMS Interview Questions and Answers
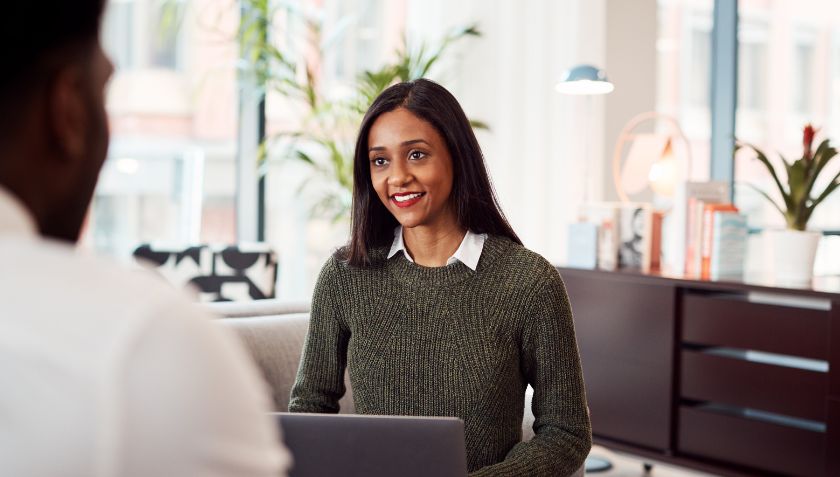
Interview Questions For Selenium with Java
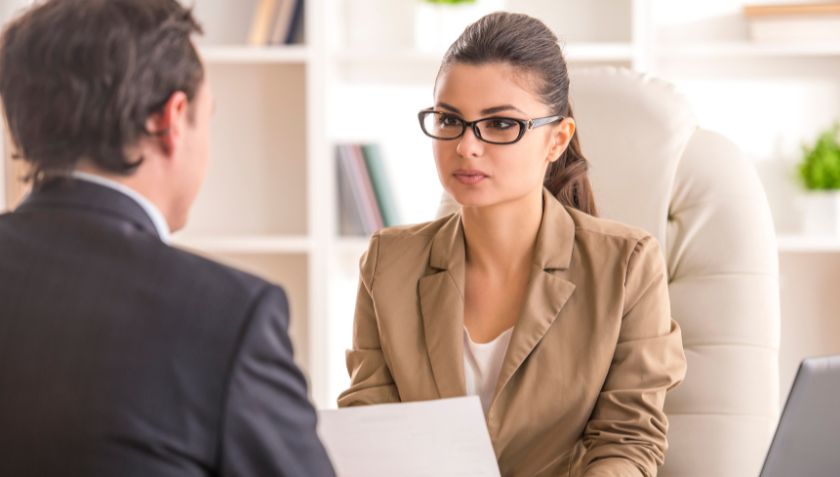
No Comments Yet
Let us know what you think