Node JS Interview Questions
Q1. What is node.js?
Ans: Node.js is a Server side scripting which is used to build scalable programs. Its multiple advantages over other server side languages, the prominent being non-blocking I/O.
Q2. Where can we use node.js?
Node.js can be used for the following purposes
- Web applications ( especially real-time web apps )
- Network applications
- Distributed systems
- General purpose applications
Q3. What is the advantage of using node.js?
Ans: It provides an easy way to build scalable network programs
- Generally fast
- Great concurrency
- Asynchronous everything
- Almost never blocks
Q4. What are the two types of API functions in Node.js ?
The two types of API functions in Node.js are
- Asynchronous, non-blocking functions
- Synchronous, blocking functions
Q5. What is control flow function?
Ans: A generic piece of code which runs in between several asynchronous function calls is known as control flow function.
Q6. Why Node.js is single threaded?
Ans: For async processing, Node.js was created explicitly as an experiment. It is believed that more performance and scalability can be achieved by doing async processing on a single thread under typical web loads than the typical thread based implementation.
Q7. Can you access DOM in node?
Ans: No, you cannot access DOM in node.
Q8. Using the event loop what are the tasks that should be done asynchronously?
Ans:
- I/O operations
- Heavy computation
- Anything requiring blocking
Q9. Why node.js is quickly gaining attention from JAVA programmers?
Ans: Node.js is quickly gaining attention as it is a loop based server for JavaScript. Node.js gives user the ability to write the JavaScript on the server, which has access to things like HTTP stack, file I/O, TCP and databases.
Q10. What is ‘Callback’ in node.js?
Ans: Callback function is used in node.js to deal with multiple requests made to the server. Like if you have a large file which is going to take a long time for a server to read and if you don’t want a server to get engage in reading that large file while dealing with other requests, call back function is used. Call back function allows the server to deal with pending request first and call a function when it is finished.Refer our Node.js Course for an extra information.
Q11. Mention the framework most commonly used in node.js?
Ans: “Express” is the most common framework used in node.js
Q12. What is an event loop in Node.js ?
Ans: To process and handle external events and to convert them into callback invocations an event loop is used. So, at I/O calls, node.js can switch from one request to another .
Q13. Mention the steps by which you can async in Node.js?
Ans: By following steps you can async Node.js
- First class functions
- Function composition
- Callback Counters
- Event loops
Q14. What are the pros and cons of Node.js?
Ans:
Pros:
- If your application does not have any CPU intensive computation, you can build it in Javascript top to bottom, even down to the database level if you use JSON storage object DB like MongoDB.
- Crawlers receive a full-rendered HTML response, which is far more SEO friendly rather than a single page application or a websockets app run on top of Node.js.
Cons:
- Any intensive CPU computation will block node.js responsiveness, so a threaded platform is a better approach.
- Using relational database with Node.js is considered less favourable
Q15. How Node.js overcomes the problem of blocking of I/O operations?
Ans: Node.js solves this problem by putting the event based model at its core, using an event loop instead of threads.
Q16. What is the difference between Node.js vs Ajax?
Ans: The difference between Node.js and Ajax is that, Ajax (short for Asynchronous Javascript and XML) is a client side technology, often used for updating the contents of the page without refreshing it. While,Node.js is Server Side Javascript, used for developing server software. Node.js does not execute in the browser but by the server.
Q17. What are the Challenges with Node.js ?
Ans: Emphasizing on the technical side, it’s a bit of challenge in Node.js to have one process with one thread to scale up on multi core server.
Q18. What does it mean “non-blocking” in node.js?
Ans: In node.js “non-blocking” means that its IO is non-blocking. Node uses “libuv” to handle its IO in a platform-agnostic way. On windows, it uses completion ports for unix it uses epoll or kqueue etc. So, it makes a non-blocking request and upon a request, it queues it within the event loop which call the JavaScript ‘callback’ on the main JavaScript thread.
Q19. What is the command that is used in node.js to import external libraries?
Ans: Command “require” is used for importing external libraries, for example, “var http=require (“http”)”. This will load the http library and the single exported object through the http variable.
Q20. What's your favourite HTTP framework and why?
Ans: There is no right answer for this. The goal here is to understand how deeply one knows the framework she/he uses, if can reason about it, knows the pros, cons.
Q21. What's the difference between operational and programmer errors?
Ans: Operation errors are not bugs, but problems with the system, likerequest timeout or hardware failure.
On the other hand programmer errors are actual bugs.
Q22. What is the latest version of Node.js available?
Ans: Latest version of Node.js is - v0.10.36.
Q23. What are the features of Node.js?
Ans: Below are the features of Node.js –
- Very Fast
- Event driven and Asynchronous
- Single Threaded but highly Scalable
Q24. Explain REPL in Node.js?
Ans: REPL stands for Read Eval Print Loop. Node.js comes with bundled REPL environment which performs the following desired tasks –
- Eval
- Loop
- Read
Q25. Explain variables in Node.js?
Ans: Variables are used to store values and print later like any conventional scripts. If “var” keyword is used then value is stored in variable. You can print the value in the variable using - console.log().
Eg:
$ node
> a = 30
30
> var b = 50
undefined
> a + b
80
> console.log("Hi")
Hi
undefined
Q26. List out some REPL commands in Node.js?
Ans: Below are the list of REPL commands –
- Ctrl + c - For terminating the current command.
- Ctrl + c twice – For terminating REPL.
- Ctrl + d - For terminating REPL.
- Tab Keys - list of all the current commands.
- .break - exit from multiline expression.
- .save with filename - save REPL session to a file.
Q27. Mention the command to stop REPL in Node.js?
Ans: Command - ctrl + c twice is used to stop REPL.
Q28. Explain NPM in Node.js?
Ans: NPM stands for Node Package Manager (npm) and there are two functionalities which NPM takes care of mainly and they are –
- Online repositories for node.js modules or packages, which can be searched on search.nodejs.org
- Dependency Management, Version Management and command line utility for installing Node.js packages.
Q29. Mention command to verify the NPM version in Node.js?
Ans: Below command can be used to verify the NPM version –
$ npm --version
Q30. How you can update NPM to new version in Node.js?
Ans: Below commands can be used for updating NPM to new version –
$ sudo npm install npm -g
/usr/bin/npm -> /usr/lib/node_modules/npm/bin/npm-cli.js
npm@2.7.1 /usr/lib/node_modules/npm
Q31. Explain callback in Node.js?
Ans: Callback is called once the asynchronous operation has been completed. Node.js heavily uses callbacks and all API’s of Node.js are written to support callbacks.
Q32. How Node.js can be made more scalable?
Ans: Node.js works good for I/O bound and not CPU bound work. For instance if there is a function to read a file, file reading will be started during that instruction and then it moves onto next instruction and once the I/O is done or completed it will call the callback function. So there will not be any blocking.
Q33. Explain global installation of dependencies?
Ans: Globally installed dependencies or packages are stored in <user-directory>/npm directory and these dependencies can be used in Command Line Interface function of any node.js.
Q34. What you mean by chaining in Node.JS?
Ans: It’s a mechanism in which output of one stream will be connected to another stream and thus creating a chain of multiple stream operations.
Q35. Explain Child process module?
Ans: Child process module has following three major ways to create child processes –
- spawn - child_process.spawn launches a new process with a given command.
- exec - child_process.exec method runs a command in a shell/console and buffers the output.
- fork - The child_process.fork method is a special case of the spawn() to create child processes.
Q36. Why to use exec method for Child process module?
Ans: “exec” method runs a command in a shell and buffers the output. Below is the command –
child_process.exec(command[, options], callback)
Q37. List out the parameters passed for Child process module?
Ans: Below are the list of parameters passed for Child Process Module –
child_process.exec(command[, options], callback)
- command - This is the command to run with space-separated arguments.
- options – This is an object array which comprises one or more following options –
- cwd
- uid
- gid
- killSignal
- maxBuffer
- encoding
- env
- shell
- timeout
callback – This is the function which is gets 2 arguments – stdout, stderr and error.
Q38. What is the use of method – “spawn()”?
Ans: This method is used to launch a new process with the given commands. Below is the method signature –
child_process.spawn(command[, args][, options])
Q39. What is the use of method – “fork()”?
Ans: This method is a special case for method- “spawn()” for creating node processes. The method signature –
child_process.fork(modulePath[, args][, options])
Q40. Explain Piping Stream?
Ans: This is a mechanism of connecting one stream to other and this is basically used for getting the data from one stream and pass the output of this to other stream.
Q41. What would be the limit for Piping Stream?
Ans: There will not be any limit for piping stream.
Q42. Explain FS module ?
Ans: Here FS stands for “File System” and fs module is used for File I/O. FS module can be imported in the following way –
var test = require("fs")
Q43. Explain “Console” in Node.JS?
Ans: “Console” is a global object and will be used for printing to stderr and stdout and this will be used in synchronous manner in case of destination is either file or terminal or else it is used in asynchronous manner when it is a pipe.
Q44. Explain – “console.log([data][, ...])” statement in Node.JS?
Ans: This statement is used for printing to “stdout” with newline and this function takes multiple arguments as “printf()”.
Q45. List out the properties of process?
Below are the useful properties of process –
- Platform
- Stdin
- Stdout
- Stderr
- execPath
- mainModule
- execArgv
- config
- arch
- title
- version
- argv
- env
- exitCode
Q46. How to concatenate buffers in NodeJS?
Ans: The syntax to concatenate buffers in NodeJS is
var MyConctBuffer = Buffer.concat([myBuffer1, myBuffer2]);
Q47. How to compare buffers in NodeJS?
Ans: To compare buffers in NodeJS, use following code –
Mybuffer1.compare(Mybuffer2);
Q48. How to copy buffers in NodeJS?
Ans: Below is the syntax to copy buffers in NodeJS –
buffer.copy(targetBuffer[, targetStart][, sourceStart][, sourceEnd])
Q49. What are the differences between “readUIntBE” and “writeIntBE” in Node.JS?
Ans:
- readUIntBE - It’s a generalized version of all numeric read methods, which supports up to 48 bits accuracy. Setting noAssert to “true” to skip the validation.
- writeIntBE - This will write the value to the buffer at the specified byteLength and offset and it supports upto 48 bits of accuracy.
Q50. Why to use “SetTimeout” in Node.JS?
Ans: This is the global function and it is used to run the callback after some milliseconds.
Syntax of this method –
setTimeout(callbackmethod, millisecs)
You May Also Like
These Related Stories
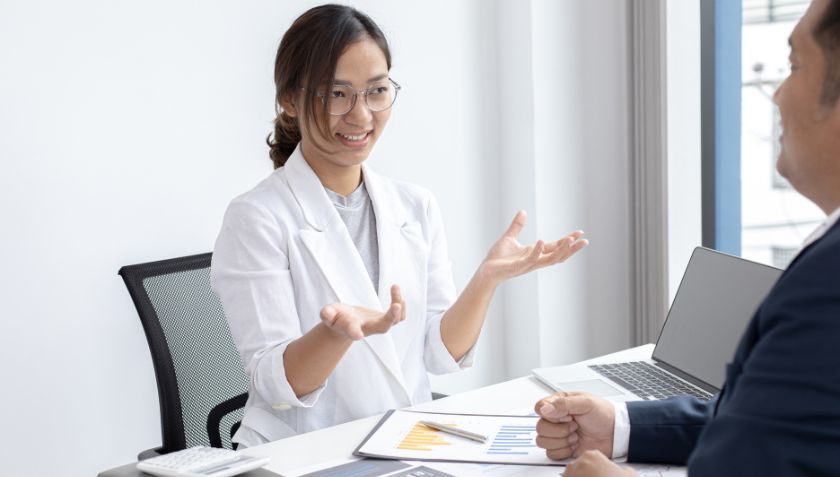
MVC Interview Questions and Answers For 2 Years Experience
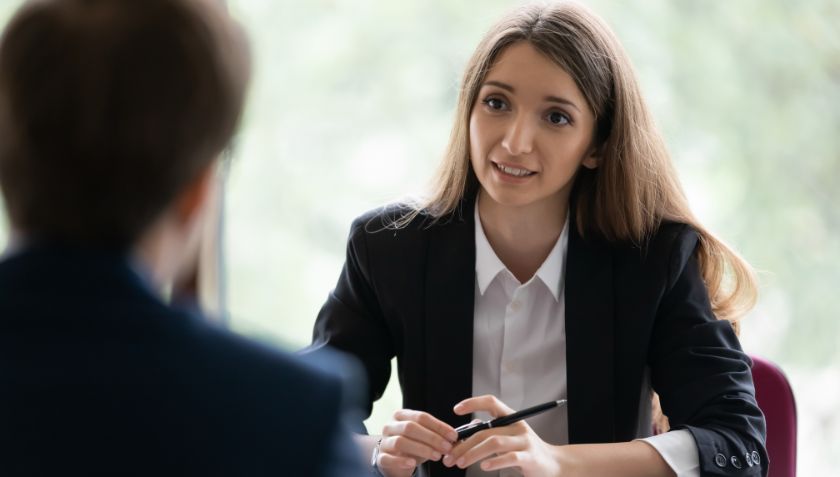
Maven Interview Questions and Answers
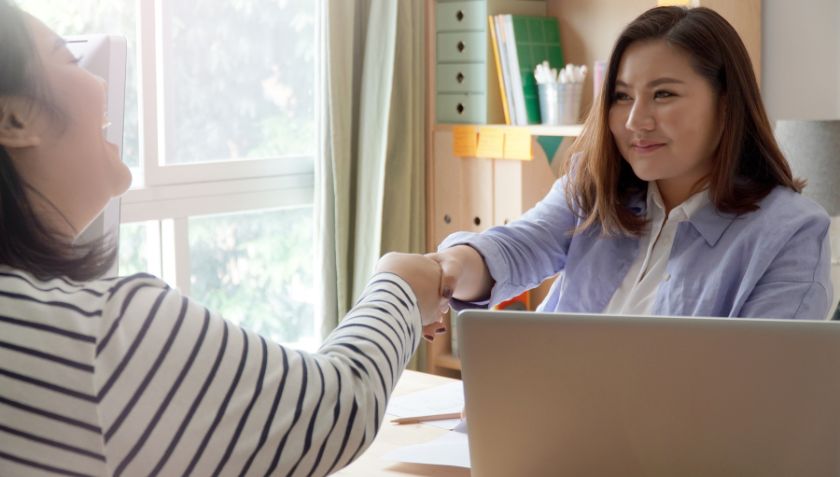
No Comments Yet
Let us know what you think