Kotlin Interview Questions and Answers
Q1. What Is Kotlin?
Ans: It is an open-source programming language that combines object-oriented programming features.
The features like Range Expression, Extension Function, Companion Object, Smart casts, Data classes are considered to be a surplus of the Kotlin Language.
Q2. Who is the developer of Kotlin?
Ans: Kotlin was developed by JetBrains.
Q3. Explain the data classes in Kotlin?
Ans: In programming, we use classes to hold data and these classes are called as data classes.
An object can be initialized in the data class and to access the individual parameters of these data classes, we use component functions.
Q4. What is the basic difference between the fold and reduce in Kotlin? When to use which?
Ans:
fold - takes an initial value, and the first invocation of the lambda you pass to it will receive that initial value and the first element of the collection as parameters.
listOf(1, 2, 3).fold(0) { sum, element -> sum + element }
The first call to the lambda will be with parameters 0
and 1
.
Having the ability to pass in an initial value is useful if you have to provide some sort of default value or parameter for your operation.
reduce - Doesn't take an initial value, but instead starts with the first element of the collection as the accumulator (called sum
in the following example)
listOf(1, 2, 3).reduce { sum, element -> sum + element }
The first call to the lambda here will be with parameters 1
and 2
.
Q5. Which Type Of Programming Does Kotlin Support?
Ans: Kotlin supports only two types of programming, and they are:
- Procedural programming
- Object-oriented programming
- Functional
Q6. Why should we use Kotlin?
Ans:
- Kotlin is concise
- Kotlin is null-safe
- Kotlin is interoperable
Q7. What Are The Different Types Of Constructors In Kotlin?
Ans: There are two types of constructors in Kotlin:
- Primary constructor: It is a section of the Class header and is declared after the class name.
- Secondary constructor: This constructor is declared inside the body.
- Note: There can be more secondary constructors for a class.
Q8. Why Use Kotlin in Mobile App Development?
Ans: Since Kotlin simplifies many syntactical elements of Java, it’s easier to write concise, well-documented code. Additionally, since it runs directly on JVM, enterprises hardly need to invest in new tech stacks. So the cost-benefit adjustment is excellent.
Moreover, Kotlin has already started to replace many Java-based Android apps, alongside iOS apps written in Swift. This number will only increase over time and adapting to Kotlin will become a must for modern enterprises. So, to stay ahead of the competition, developers should embrace Kotlin today.
Q9. What are Kotlin’s Best Features?
Ans: Some of Kotlin’s best features are-
- It supports both the object-oriented and functional programming paradigm.
- It provides easy-to-use lambda functions that are unavailable in Java.
- Maintaining Kotlin is considerably cheap and provides excellent fault tolerance.
- Allows developing Node.js applications and JVMs.
- Great support for asynchronous communication.
- Exceptional compatibility with existing Java codes.
Q10. What is Null Safety in Kotlin?
Ans: Kotlin comes with in-built protection against unwanted null references which allows it to be more fault-tolerant. It thus allows programs to reduce NullPointerExceptions during runtime and prevents unwanted program crashes. This is a common problem faced by most existing Java software and causes losses costing millions of dollars. This is often coined as Null Safety among Kotlin developers.
Q11. Mention The Structural Expressions In Kotlin?
Ans: There are three Structural expressions in Kotlin.
They are:
- Return: It returns from the nearest enclosing function or anonymous function by default.
- Break: This expression terminates the closest enclosing loop.
- Continue: This expression proceeds you to the next closest enclosing loop.
Q12. What Are The Modifiers That Are Available In Kotlin?
Ans: The modifier in Kotlin provides the developer to customize the declarations as per the requirements.
Kotlin provides four modifiers.
They are:
- Private: This makes the declaration visible only inside the file containing the declaration.
- Public: It is by default, which means that the declarations will be visible everywhere.
- Internal: This makes the declaration visible everywhere in the same modules.
- Protected: This keeps the declaration protected and is not available for top-level declarations.
Q13. What is Elvis operator in Kotlin?
Ans: In Kotlin, you can assign null values to a variable by using the null safety property. To check if a value is having null value then you can use if-else or can use the Elvis operator i.e. ?:
For example:
var name:String? = "Mindorks"
val nameLength = name?.length ?: -1
println(nameLength)
The Elvis operator(?:
) used above will return the length of name if the value is not null otherwise if the value is null, then it will return -1
.
Q14. How to convert a Kotlin source file to a Java source file?
Ans: Steps to convert your Kotlin source file to Java source file:
- Open your Kotlin project in the IntelliJ IDEA / Android Studio.
- Then navigate to Tools > Kotlin > Show Kotlin Bytecode.
- Now click on the Decompile button to get your Java code from the bytecode.
Q15 . What is the use of @JvmStatic, @JvmOverloads, and @JvmFiled in Kotlin?
Ans:
- @JvmStatic: This annotation is used to tell the compiler that the method is a static method and can be used in Java code.
- @JvmOverloads: To use the default values passed as an argument in Kotlin code from the Java code, we need to use the @jvmoverloads annotation
- @JvmField: To access the fields of a Kotlin class from Java code without using any getters and setters, we need to use the @jvmfield in the Kotlin code.
Q16. Can we use primitive types such as int, double, float in Kotlin?
Ans: In Kotlin, we can't use primitive types directly. We can use classes like Int, Double, etc. as an object wrapper for primitives. But the compiled bytecode has these primitive types.
Q17. What is the use of abstraction in Kotlin?
Ans: Abstraction is the most important concept of Objected Oriented Programming. In Kotlin, the abstraction class is used when you know what functionalities a class should have. But you are not aware of how the functionality is implemented or if the functionality can be implemented using different methods.
Q18. List the Basic data types of Kotlin?
Ans: Data types of a constant or variable decide what type of variable it is and how much space is required to store it.
The basic data types in Kotlin are:
- Numbers
- Characters
- Strings
- Arrays
- Booleans
Q19. State the advantages and disadvantages of Kotlin?
Ans:
Advantages:
Kotlin is simple and easy to learn as its syntax is similar to that of Java.
It is the functional language that is based on JVM (Java Virtual Machine), which removes the boilerplate codes. Upon all this, Kotlin is considered as an expressive language that is easily readable and understandable and the performance is substantially good.
It can be used by any desktop, web server or mobile-based applications.
Disadvantages:
Kotlin does not provide the static modifier, which causes problems for conventional java developers.
In Kotlin, the function declaration can be done in many places in the application, which creates trouble for the developer to understand which function is being called.
Q20. Explain Functions In Kotlin?
Ans: Kotlin functions are first-class functions that are easily stored in variables and data structures and can be pass as arguments and returned from other higher-order functions.
Sample function declaration and usage in Kotlin
fun double(x: Int): Int {
return 2 * x
}
val result = double(2)
Q21. List out some of the extension methods in kotlin?
Ans: Some of the extension methods are:
- read Text(): Helps to read content in the files to a single string.
- buffer Reader(): It is used to read the contents of the file to buffer the reader
- read each line(): It reads each line by line in the file
- readlines(): It helps to read lines of file for listing
Q22. What is an inline function in Kotlin?
Ans: Inline function instruct compiler to insert complete body of the function wherever that function got used in the code. To use an Inline function, all you need to do is just add an inline keyword at the beginning of the function declaration.
Q23. What is noinline in Kotlin?
Ans: While using an inline function and want to pass some lambda function and not all lambda function as inline, then you can explicitly tell the compiler which lambda it shouldn't inline.
inline fun doSomethingElse(abc: () -> Unit, noinline xyz: () -> Unit) {
abc()
xyz()
}
Q24. Is inheritance compile in Kotlin?
Ans: Formal inheritance structure does not compile in the kotlin. By using an open modifier we can finalize classes.
open class B
{
}
class c = B()
{
}
Q25. What is mean by init block?
Ans: Init is a login block and it is executed in the primary constructor and initialized. If you want to revoke in the secondary constructor then it starts working after the primary constructor in the chain form.
Q26. How to Compare Two Strings in Kotlin?
Ans: String processing comprises an essential portion of any app development. Interviewees are often asked how to handle this during Kotlin interview questions. You can use the equality operator ‘==’ to do this, as demonstrated by the following example.
val a: String = "This is the first string"
val b: String = "This is the second" + "string"
if (a == b) println("The Strings are Similar")
else println("They don't match!")
Q27. Describe For Loops in Kotlin?
Ans: Loops are a crucial programming construct that allows us to iterate over things as our program requires. Kotlin features all the commonly used loops such as for, while, and do-while. We’re describing the for loop in a nutshell in the following section.
val sports = listOf("cricket", "football", "basketball")
for (sport in sports) { // for loop
println("Let's play $sport!")
}
The above snippet illustrates the use of the for loop in Kotlin. It’s quite similar to Python and Ruby.
Q28. What is the Purpose of Object Keyword?
Ans: Kotlin provides an additional keyword called object alongside its standard object-oriented features. Contrary to the traditional object-oriented paradigm where you define a class and create as many of its instances as you require, the object keyword allows you to create a single, lazy instance. The compiler will create this object when you access it in your Kotlin program. The following program provides a simple illustration.
fun calcRent(normalRent: Int, holidayRent: Int): Unit {
val rates = object{
var normal: Int = 30 * normalRent
var holiday: Int = 30 * holidayRent
}
val total = rates.normal + rates.holiday
print("Total Rent: $$total")
}
fun main() {
calcRent(10, 2)
}
Q29. Explain the Fundamental Data Types of Kotlin?
Ans:
Kotlin data types define the procedures available on some data. The compiler allocates memory space for variables using their data type. Like many popular programming languages, Kotlin features some often-used data types. Take a look at the below section for a short overview of various Kotlin data types.
- integers – contrary to Python, Kotlin has a limited size for integers; available integer types are Long, Int, Short, and Byte
- floats – floating-point values contain fractional values; they can be declared using Float or Double
- characters – represented by the Char modifier; usually hold a single Unicode character
- strings – they are created using the String type and are immutable like in Java
- booleans – represents the boolean values true and false
- arrays – arrays in Kotlin are represented using the Array class
Q30. How do String Interpolations Work in Kotlin?
Ans: String interpolations work with multiple placeholders and first evaluate their value to display the final string output. This final output will contain the corresponding values of the placeholders. The below code snippet will illustrate a simple example of Kotlin string interpolation.
fun main(args: Array<String>) { // String Interpolation
print("Please enter your name here:")
val name:String? = readLine()
print("Hello, $name!")
}
Here, the Kotlin compiler first receives the user input and interpolates this value in place of the placeholder $name. The last line of the snippet is translated by the compiler as shown below –
new StringBuilder().append("Hello, ").append(name).append("!").toString()
You May Also Like
These Related Stories
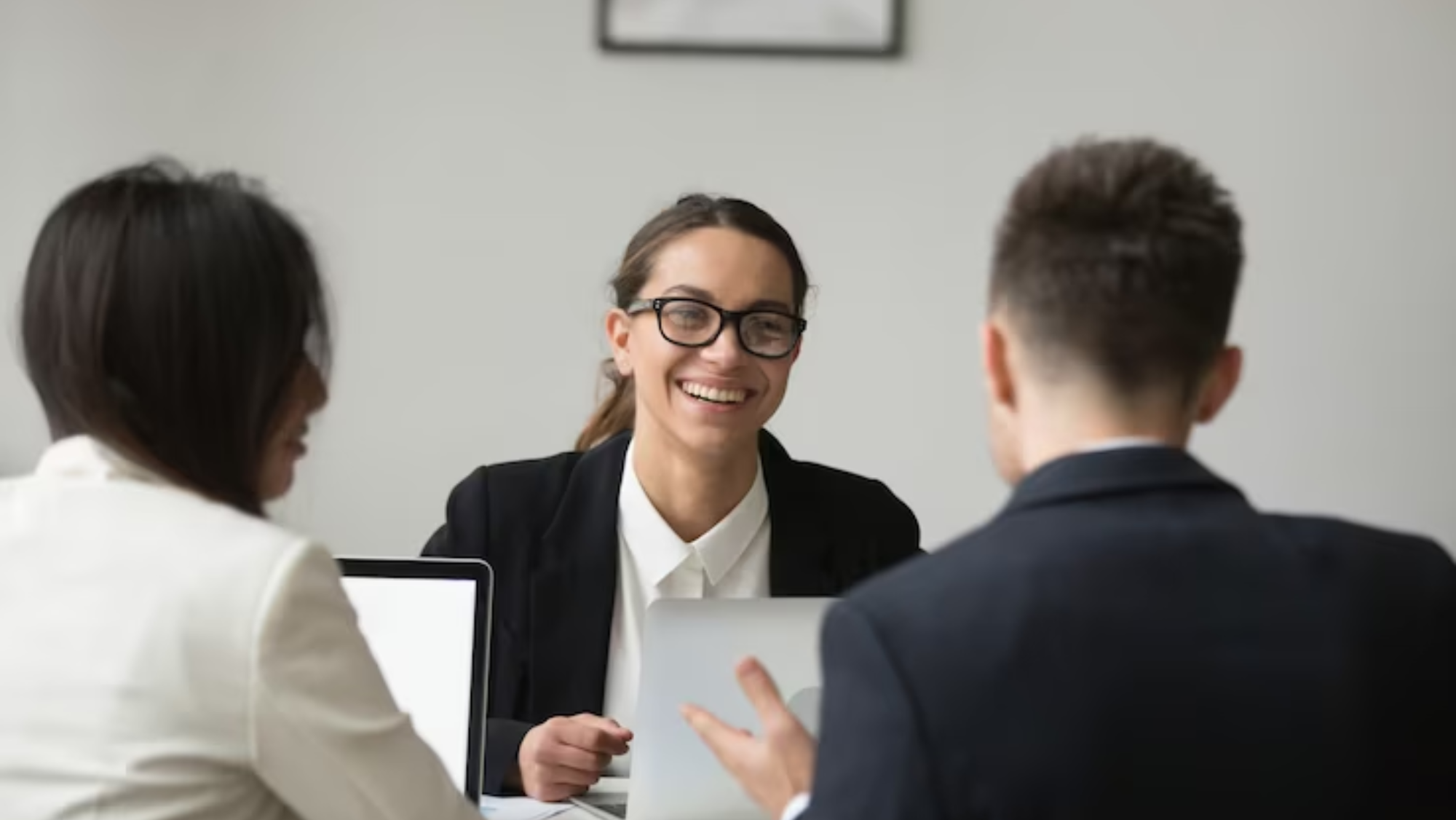
Kotlin Interview Questions And answers
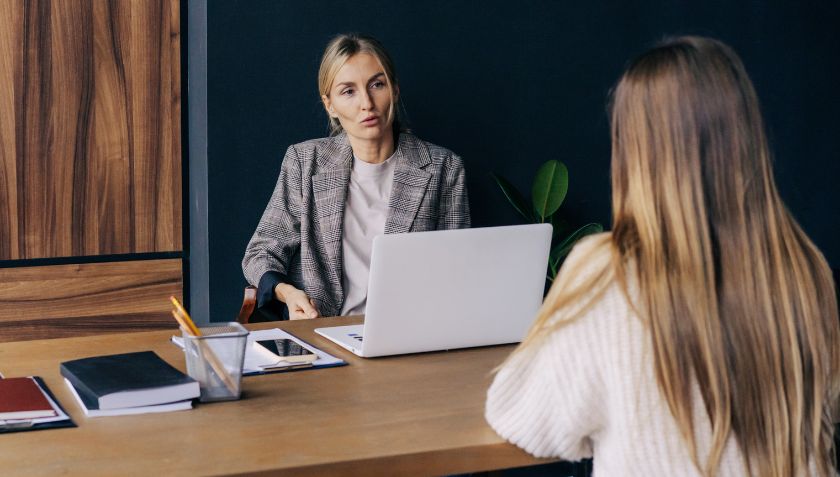
KnockoutJS Interview Questions and Answers
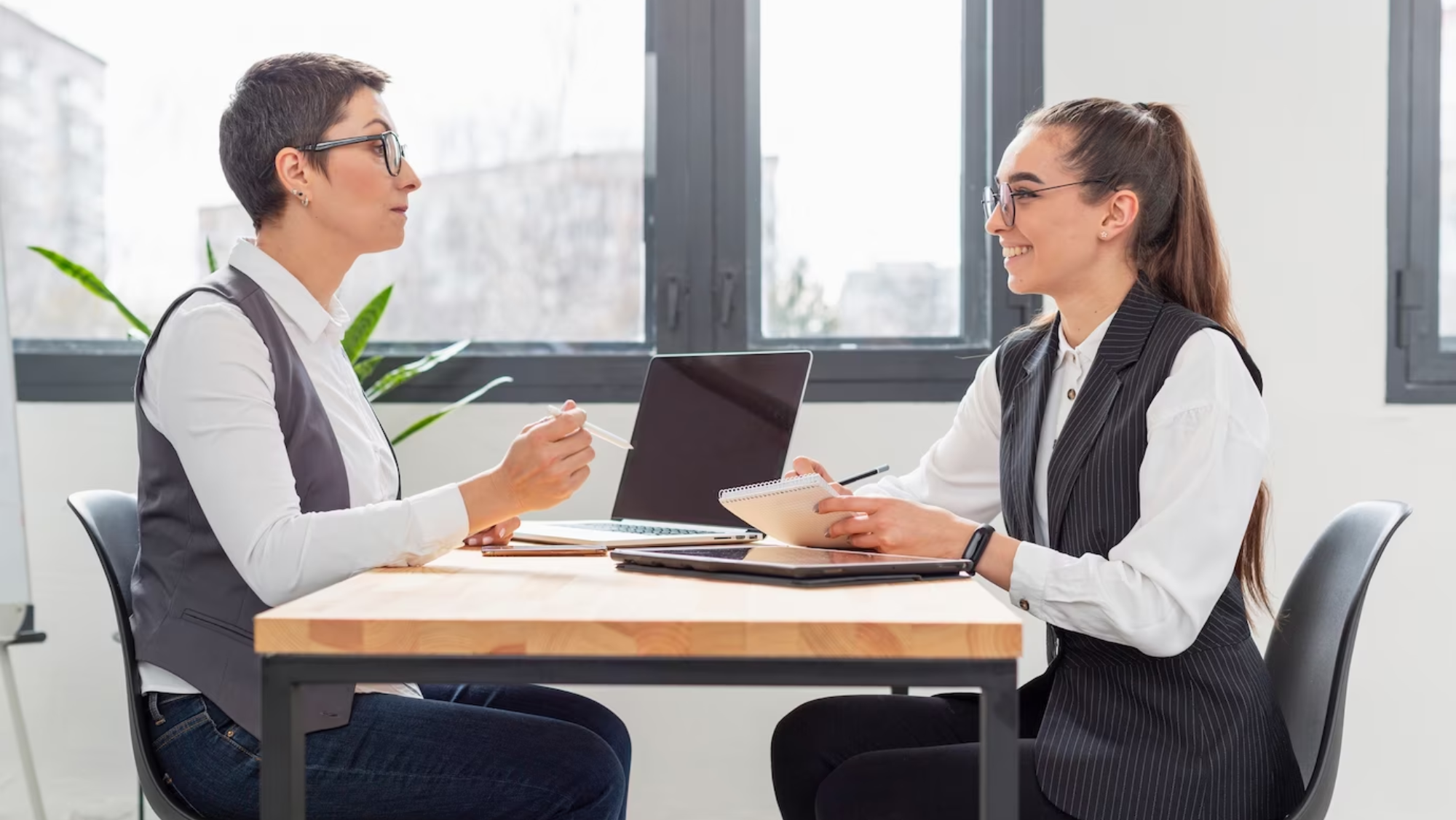
No Comments Yet
Let us know what you think