Flutter Interview Questions And Answers
1. What is Flutter?
Ans: Flutter is an open-source UI framework developed by Google for building natively compiled applications for mobile, web, and desktop using a single codebase.
2. What are the advantages of Flutter?
Ans: Flutter offers advantages like hot reload for faster development, a rich set of customizable widgets, excellent performance, cross-platform compatibility, and a growing community.
3. What's the difference between hot reload and hot restart in Flutter?
Ans: Hot reload injects the updated code into the running app, maintaining the app's state. Hot restart restarts the app from scratch, losing the current state.
4. Explain the widget tree in Flutter.
Ans: The widget tree represents the hierarchical structure of widgets in a Flutter app. It defines the UI and layout, with each widget having its properties and child widgets.
5. What is the difference between Stateless Widget and Stateful Widget?
Ans: Stateless Widget is immutable and doesn't have any internal state, while Stateful Widget can change its state dynamically, making it suitable for components with changing data.
6. How do you handle state management in Flutter?
Ans: Flutter offers multiple state management approaches, such as setState for simple cases, Provider for dependency injection, and BLoC pattern or Redux for more complex apps.
7. What is a key in Flutter?
Ans: A key is an identifier used to uniquely identify and track widgets. Keys are crucial when working with dynamic lists or updating the state of specific widgets.
8. How does Flutter achieve high-performance UI rendering?
Ans: Flutter uses Skia, a 2D graphics library, to directly render UI components to the screen, resulting in fast and smooth UI performance.
9. What is the purpose of the pubspec.yaml file?
Ans: The pubspec.yaml file is used to declare dependencies, specify assets like images or fonts, configure the app, and define metadata for a Flutter project.
10. How do you handle navigation between screens in Flutter?
Ans: Flutter provides the Navigator class to manage navigation between screens. You can use methods like Navigator.push and Navigator.pop to navigate and pass data between screens.
11. What is the purpose of the initState() method in StatefulWidget?
Ans: The initState() method is called when a StatefulWidget is inserted into the widget tree. It allows you to initialize the state of the widget before it is displayed.
12. How do you handle form validation in Flutter?
Ans: Flutter provides validators like RequiredValidator and RegExpValidator, which can be used with TextFormField to validate user input. You can also implement custom validation logic.
13. What are keys used for in Flutter?
Ans: Keys are used to uniquely identify and track widgets, especially when widgets are dynamically added or removed from the widget tree. They help Flutter efficiently update the UI.
14. How can you make HTTP requests in Flutter?
Ans: Flutter offers packages like http and dio for making HTTP requests. You can use methods like get(), post(), put(), and delete() to interact with RESTful APIs.
15. Explain the concept of asynchronous programming in Flutter.
Ans: Asynchronous programming in Flutter allows tasks to run concurrently, avoiding blocking the main thread. It is commonly used when making API calls or performing time-consuming operations.
16. How can you handle platform-specific code in Flutter?
Ans: Flutter provides platform channels, allowing communication between Flutter and native code. You can use MethodChannels to invoke platform-specific functionality and receive results.
17. What is the purpose of the setState() method?
Ans: The setState() method is used to update the state of a StatefulWidget. When called, it triggers a rebuild of the widget, ensuring the UI reflects the updated state.
18. How do you handle user input in Flutter?
Ans: Flutter provides various input widgets like TextField and GestureDetector to handle user interactions. You can listen for events like onTap, onChanged, or onSubmitted to respond to user input.
19. Explain the concept of widget composition in Flutter.
Ans: Widget composition in Flutter involves combining multiple smaller widgets to create complex UI components. It enables code reuse, modularity, and building custom UI elements.
20. How can you handle errors and exceptions in Flutter?
Ans: In Flutter, you can handle errors and exceptions using try-catch blocks. For asynchronous operations, you can use async/await and handle exceptions using try-catch or onError callbacks.
21. What are keys used for in Flutter?
Ans: Keys are used to uniquely identify and track widgets, especially when widgets are dynamically added or removed from the widget tree. They help Flutter efficiently update the UI.
22. How do you handle app localization in Flutter?
Ans: Flutter provides the flutter_localizations package for app localization. You can define different language resource files and use the Intl package to handle translations.
23. How can you implement animations in Flutter?
Ans: Flutter offers several animation classes like Animation, Tween, and AnimatedBuilder. You can use these to create animations by defining animation controllers, interpolations, and transitions.
24. What is the purpose of the SingleTickerProviderStateMixin?
Ans: The SingleTickerProviderStateMixin is used to provide a ticker that notifies when frames are ready for animations. It's commonly used with AnimationController to animate widgets.
25. How do you test Flutter applications?
Ans: Flutter provides a rich testing framework. You can write unit tests using the test package, widget tests to test UI components, and integration tests using the flutter_test package.
You May Also Like
These Related Stories
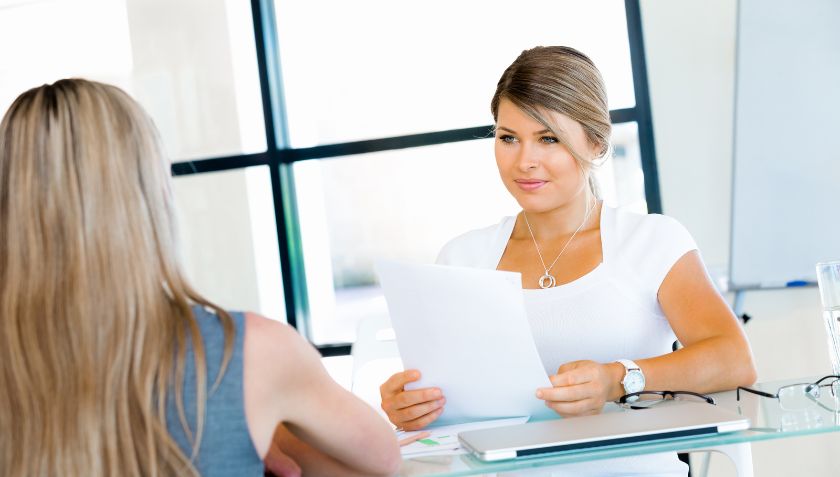
Flutter and Dart Interview Questions and Answers
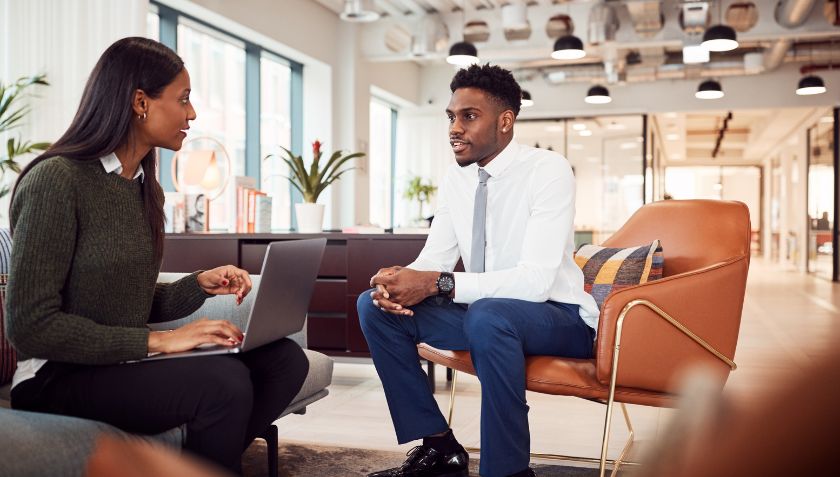
Angular js Interview Questions
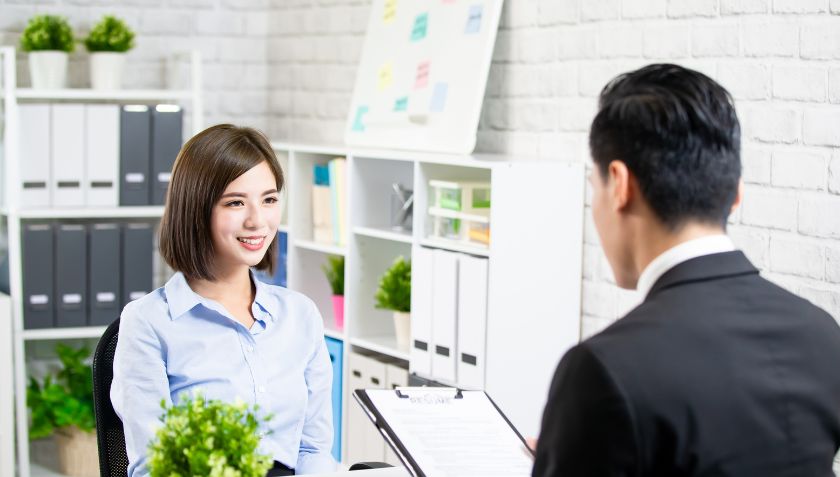
No Comments Yet
Let us know what you think