Embedded Systems Interview Questions of 2018
Q1. What Is Pass by Value and Pass by Reference? How Are Structure Passed As Arguments?
The parameter to a function can be a copy of a value that is represented by variable or can be a reference to a memory space that stores value of variable. The former is referred to as pass by value and the latter is referred to as pass by reference. The difference is that when parameters are passed by value the changes made to the variable value within the function is not reflected in the caller function, but when passed as reference changes are reflected outside the called function. The structures are always passed by reference.
Q2. What Is The Volatile Keyword Used For?
The volatile keyword is used to represent variables that point to memory in other mapped devices. In such a case the value of the variable can be changed outside of a program. The compiler does not do additional optimizations to the code if there is volatile keyword.
Q3. What Is A Semaphore? What Are The Different Types Of Semaphore?
The semaphore is an abstract data store that is used to control resource accesses across the various threads of execution or across different processes. There are two types of semaphores:
- The binary semaphore which can take only 0, 1 values. (used when there is contention for a single resource entity).
- The counting semaphore which can take incremental values to certain limit (used when number of resources is limited)
Q4. What Is Meant By A Forward Reference In C?
The forward reference refers to the case when we point an address space of a smaller data type with a pointer of a bigger data type. This can be pictured as allocating memory in single bytes and accessing it with integer pointer as chunks of 4.
Q5. What Does Malloc Do? What Will Happen If We Have A Statement Like Malloc(sizeof(0));
Malloc is the function that is used for dynamically allocating memory to the different variables. The malloc returns a memory pointer of void type (void *). The statement malloc(sizeof(0)) returns a valid integer pointer because sizeof(0) represents the size of memory taken up by the integer value of 0. The memory allocated by memory is not automatically cleaned up by the compiler after execution of the functions and should be cleaned up by the programmer using the free () function.
Q6. What Is Difference Between Using A Macro And Inline Function?
The macro are just symbolic representations and cannot contain data type differentiations within the parameters that we give. The inline functions can have the data types too defined as a part of them. The disadvantage in using both is that the inclusion of condition checks may lead to increase in code space if the function is called many times.
Q7. What Are Hard and Soft Real Time Systems?
The hard real time Embedded Systems are the one that depend on the output very strictly on time. Any late response or delay cannot be tolerated and will always be considered a failure. The soft real time systems on the other are not very rigid as the hard real time systems. The performance of the system degrades with the lateness of response, but it is bearable and can be optimized to a certain level for reuse of the result.
Q8. What Are Recursive Functions? Can We Make Them Inline?
The recursive functions refer to the functions which make calls to it before giving out the final result. These can be declared as in-line functions and the compiler will allocate the memory space intended for the first call of the function.
Q9. What Is The Size Of The Int, Char And Float Data Types?
The size of the char and int are always dependent on the underlying operating system or firmware. This is limited to the number of address lines in the address bus. The int usually takes up a value of 2 bytes or 4 bytes. The char can take up a space of 1 or 2 bytes. The float data type takes up a value of 4 bytes.
Q10. What Is The Use Of Having The Const Qualifier?
The const qualifier identifies a specific parameter or variable as read-only attribute to the function or to the entire program. This can come in handy when we are dealing with static data inside function and in a program.
Q11. Can A Pointer Be Volatile?
Yes, although this is not very common. An example is when an interrupt service routine modifies a pointer to a buffer.
Q12. What Is Loop Unrolling?
Small loops can be unrolled for higher performance, with the disadvantage of increased code size. When a loop is unrolled, a loop counter needs to be updated less often and fewer branches are executed. If the loop iterates only a few times, it can be fully unrolled, so that the loop overhead completely disappears.
Q13. What Is The Order Of Calling For The Constructors And Destructors In Case Of Objects Of Inherited Classes?
The constructors are called with base class first order and the destructors are called in the child first order. That is, the if we have 2 levels of inheritance A (base)-> B (inherit 1)-> C (inherit 2) then the constructor A is called first followed by B and C. The C destructor is called first followed by B and A.
Q14. What Typecast Is Applied When We Have A Signed And An Unsigned Int In An Expression?
The unsigned int is typecast into the signed value.
Q15. How Are Variables Mapped Across To The Various Memories By The C Compiler?
The compiler maintains the symbol table which has the related information of all the variable names along with the length of the allocated space, the access unit length for the pointer (type of pointer) and the starting address of the memory space.
Q16. What Is A Memory Leak? What Is A Segmentation Fault?
The memory leak refers to the uncleared memory may builds up across lifetime of the process. When it comes to a huge value system stalls its execution due to unavailability of the memory. The segmentation fault on the other hand refers to condition when our program tries to access a memory space that has already been freed up.
Q17. What Are Little Endian And Big Endian Types Of Storage? How Can You Identify Which Type Of Allocation A System Follows?
The little endian memory representation allocates the least address to the least significant bit and the big endian is where the highest significant bit takes up the least addressed memory space. We can identify the system’s usage by defining an integer value and accessing it as a character.
int p=0x2;
if(* (char *) &p == 0x2) printf (“little endiann”);
else printf (“big endiann”);
Q18. Explain The Properties Of A Object Oriented Programming Language.
- Encapsulation: The data that are related to the specific object are contained inside the object structure and hidden from the other entities of the environment.
- Polymorphism: The mechanism by which the same pointer can refer to different types of objects, which are basically linked by some generic commonality.
- Abstraction: Hiding the data and implementation details from the real objects. The framework of reference is still present to be used by the other objects.
- Inheritance: The way to take out the common features and have them as separate object entities only to be reused by the other objects in a modular fashion.
Q19. What Do You Mean By Interrupt Latency?
Interrupt latency refers to the time taken for the system to start the handler for the specific interrupt. The time from the time of arrival of interrupt to the time it is being handled.
Q20. What Is The Scope Of A Function That Is Declared As Static?
The static function when declared within a specific module is scoped only in that module and can only be accessed from it.
Q21. What does DMA address will deal with?
DMA address deals with physical addresses. It is a device which directly drives the data and address bus during data transfer. So, it is purely physical address.
Q22. Mention what are buses used for communication in embedded system?
For embedded system, the buses used for communication includes
- I2C: It is used for communication between multiple ICs
- CAN: It is used in automobiles with centrally controlled network
- USB: It is used for communication between CPU and devices like mouse, etc.
While ISA, EISA, PCI are standard buses for parallel communication used in PCs, computer network devices, etc.
Q23. Why embedded system is useful?
With embedded system, it is possible to replace dozens or even more of hardware logic gates, input buffers, timing circuits, output drivers, etc. with a relatively cheap microprocessor.
A watchdog timer is an electronic device or electronic card that execute specific operation after certain time period if something goes wrong with an electronic system.
Q25. Explain what is semaphore?
A semaphore is an abstract datatype or variable that is used for controlling access, by multiple processes to a common resource in a concurrent system such as multiprogramming operating system. Semaphores are commonly used for two purposes
- To share a common memory space
- To share access to files
Q26. When one must use recursion function? Mention what happens when recursion functions are declared inline?
Recursion function can be used when you are aware of the number of recursive calls is not excessive. Inline functions property says whenever it will called, it will copy the complete definition of that function. Recursive function declared as inline creates the burden on the compilers execution.
Q27. Explain what is the need for an infinite loop in embedded systems?
Embedded systems require infinite loops for repeatedly processing or monitoring the state of the program. For instance, the case of a program state continuously being verified for any exceptional errors that might just happen during run-time such as memory outage or divide by zero, etc.
Q28. List out some of the commonly found errors in Embedded Systems?
Some of the commonly found errors in embedded systems are
- Damage of memory devices static discharges and transient current
- Address line malfunctioning due to a short in circuit
- Data lines malfunctioning
- Due to garbage or errors some memory locations being inaccessible in storage
- Inappropriate insertion of memory devices into the memory slots
- Wrong control signals
Q29. Explain what are real-time embedded systems?
Real-time embedded systems are computer systems that monitor, respond or control an external environment. This environment is connected to the computer system through actuators, sensors, and other input-output interfaces.
Q30. List out various uses of timers in embedded system?
Timers in embedded system are used in multiple ways
- Real Time Clock (RTC) for the system
- Initiating an event after a preset time delay
- Initiating an even after a comparison of preset times
- Capturing the count value in timer on an event
- Between two events finding the time interval
- Time slicing for various tasks
- Time division multiplexing
- Scheduling of various tasks in RTOS
You May Also Like
These Related Stories
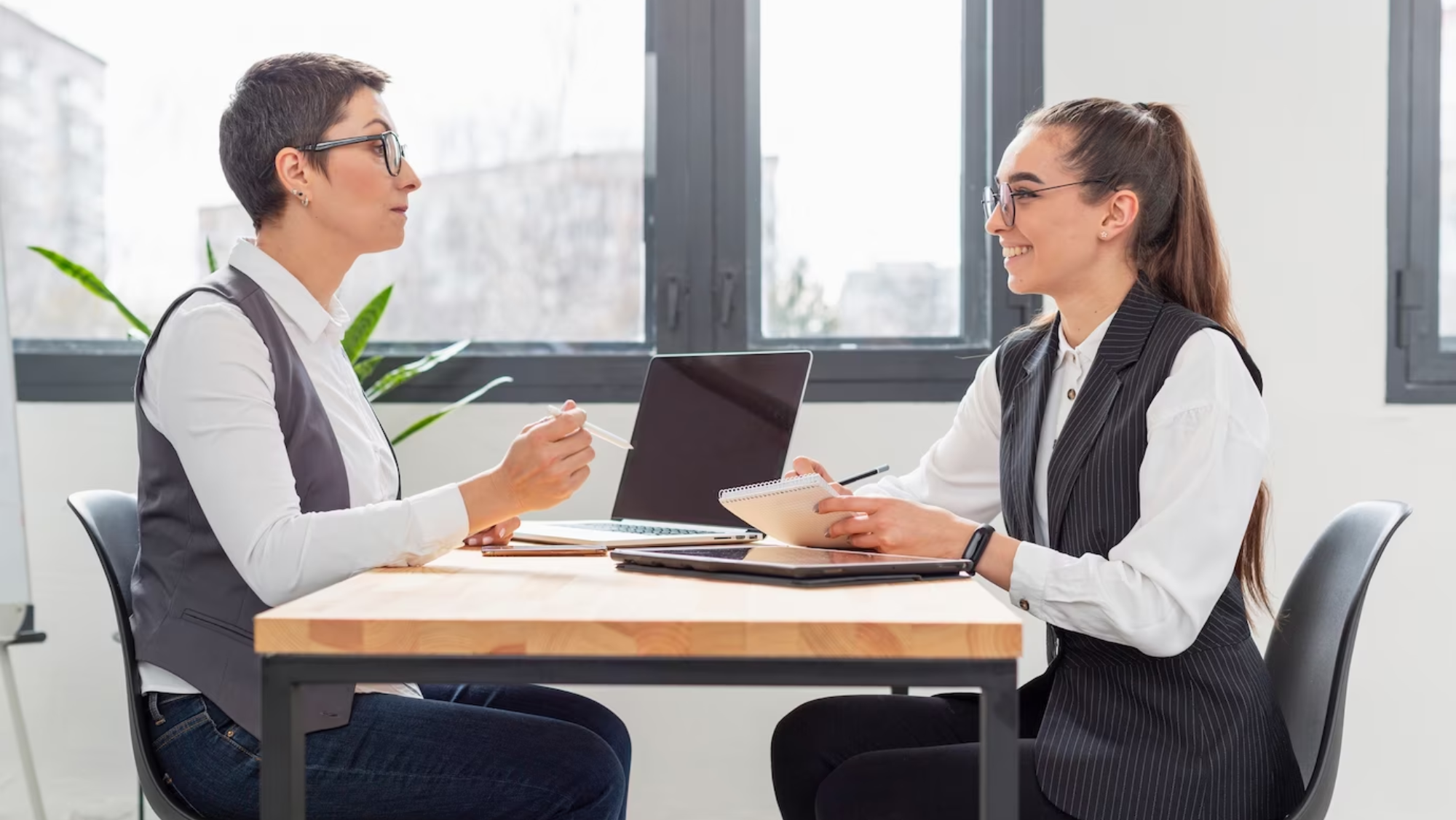
Top 25 Snowflake Interview Questions and Answers
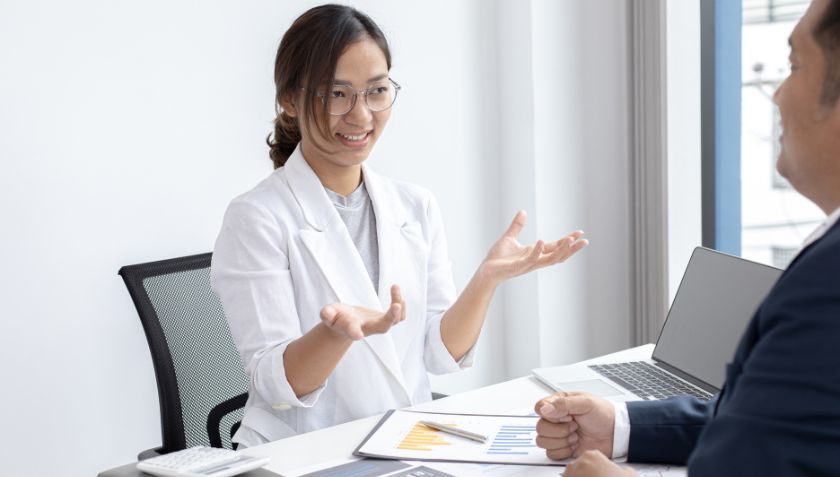
Ajax Interview Questions and Answers
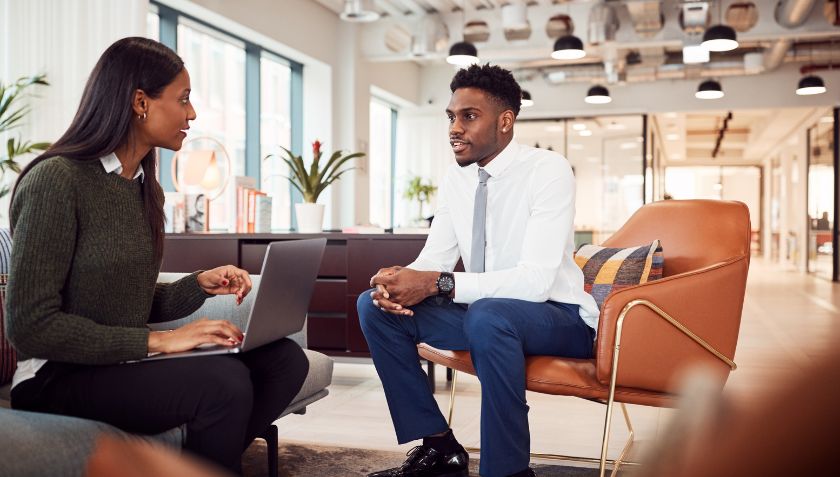
No Comments Yet
Let us know what you think