Angular JS Interview Questions and Answers
1.What is AngularJS?
AngularJS is a javascript framework used for creating single web page applications. It allows you to use HTML as your template language and enables you to extend HTML’s syntax to express your application’s components clearly
2.Explain what are the key features of AngularJS ?
The key features of AngularJS are
- Scope
- Controller
- Model
- View
- Services
- Data Binding
- Directives
- Filters
- Testable
3.Explain what is scope in AngularJS ?
Scope refers to the application model, it acts like glue between application controller and the view. Scopes are arranged in hierarchical structure and impersonate the DOM ( Document Object Model) structure of the application. It can watch expressions and propagate events.
4.Explain what is services in AngularJS ?
In AngularJS services are the singleton objects or functions that are used for carrying out specific tasks. It holds some business logic and these function can be called as controllers, directive, filters and so on.
5.Explain what is Angular Expression? Explain what is key difference between angular expressions and JavaScript expressions?
Like JavaScript, Angular expressions are code snippets that are usually placed in binding such as
The key difference between the JavaScript expressions and Angular expressions
- Context :In Angular, the expressions are evaluated against a scope object, while the Javascript expressions are evaluated against the global window
- Forgiving:In Angular expression evaluation is forgiving to null and undefined, while in Javascript undefined properties generates TypeError or ReferenceError
- No Control Flow Statements:Loops, conditionals or exceptions cannot be used in an angular expression
- Filters:To format data before displaying it you can use filters
6.With options on page load how you can initialize a select box ?
You can initialize a select box with options on page load by using ng-init directive
- <div ng-controller = “ apps/dashboard/account ” ng-switch
- On = “! ! accounts” ng-init = “ loadData ( ) ”>
7.Explain what are directives ? Mention some of the most commonly used directives in AngularJS application ?
A directive is something that introduces new syntax, they are like markers on DOM element which attaches a special behavior to it. In any AngularJS application, directives are the most important components.
Some of the commonly used directives are ng-model, ng-App, ng-bind, ng-repeat , ng-show etc.
8.Mention what are the advantages of using AngularJS ?
AngularJS has several advantages in web development.
- AngularJS supports MVC pattern
- Can do two ways data binding using AngularJS
- It has per-defined form validations
- It supports both client server communication
- It supports animations
9.Explain what Angular JS routes does ?
Angular js routes enable you to create different URLs for different content in your application. Different URLs for different content enables user to bookmark URLs to specific content. Each such bookmarkable URL in AngularJS is called a route
A value in Angular JS is a simple object. It can be a number, string or JavaScript object. Values are typically used as configuration injected into factories, services or controllers. A value should be belong to an AngularJS module.
Injecting a value into an AngularJS controller function is done by adding a parameter with the same name as the value
10.Explain what is data binding in AngularJS ?
Automatic synchronization of data between the model and view components is referred as data binding in AngularJS. There are two ways for data binding
- Data mining in classical template systems
- Data binding in angular templates
- What makes AngularJS better ?
- Registering Callbacks:There is no need to register callbacks . This makes your code simple and easy to debug.
- Control HTML DOM programmatically: All the application that are created using Angular never have to manipulate the DOM although it can be done if it is required
- Transfer data to and from the UI: AngularJS helps to eliminate almost all of the boiler plate like validating the form, displaying validation errors, returning to an internal model and so on which occurs due to flow of marshalling data
- No initilization code: With AngularJS you can bootstrap your app easily using services, which auto-injected into your application in Guice like dependency injection style.
11.What is dependency injection and how does it work?
AngularJS was designed to highlight the power of dependency injection, a software design pattern that places an emphasis on giving components their dependencies instead of hard coding them within the component. For example, if you had a controller that needed to access a list of customers, you would store the actual list of customers in a service that can be injected into the controller instead of hardcoding the list of customers into the code of the controller itself. In AngularJS you can inject values, factories, services, providers, and constants
12.Explain what is string interpolation in Angular.js ?
In Angular.js the compiler during the compilation process matches text and attributes using interpolate service to see if they contains embedded expressions. As part of normal digest cycle these expressions are updated and registered as watches.
13. Mention the steps for the compilation process of HTML happens?
Compilation of HTML process occurs in following ways
- Using the standard browser API, first the HTML is parsed into DOM
- By using the call to the $compile () method, compilation of the DOM is performed. The method traverses the DOM and matches the directives.
- Link the template with scope by calling the linking function returned from the previous step
14. Explain what is directive and Mention what are the different types of Directive?
During compilation process when specific HTML constructs are encountered a behaviour or function is triggered, this function is referred as directive. It is executed when the compiler encounters it in the DOM.
Different types of directives are
- Element directives
- Attribute directives
- CSS class directives
- Comment directives
15. Explain what is linking function and type of linking function?
Link combines the directives with a scope and produce a live view. For registering DOM listeners as well as updating the DOM, link function is responsible. After the template is cloned it is executed.
- Pre-linking function: Pre-linking function is executed before the child elements are linked. It is not considered as the safe way for DOM transformation.
- Post linking function: Post linking function is executed after the child elements are linked. It is safe to do DOM transformation by post-linking function
16.Explain what is injector?
An injector is a service locator. It is used to retrieve object instances as defined by provider, instantiate types, invoke methods and load modules. There is a single injector per Angular application, it helps to look up an object instance by its name.
17. Explain what is the difference between link and compile in Angular.js?
- Compile function: It is used for template DOM Manipulation and collect all of the directives.
- Link function: It is used for registering DOM listeners as well as instance DOM manipulation. It is executed once the template has been cloned.
18. Explain what is factory method in AngularJS?
For creating the directive, factory method is used. It is invoked only once, when compiler matches the directive for the first time. By using $injector.invoke the factory method is invoked.
19. Mention what are the styling form that ngModel adds to CSS classes ?
ngModel adds these CSS classes to allow styling of form as well as control
- ng- valid
- ng- invalid
- ng-pristine
- ng-dirty
- Mention what are the characteristics of “Scope”?
- To observer model mutations scopes provide APIs ($watch)
- To propagate any model changes through the system into the view from outside of the Angular realm
- A scope inherits properties from its parent scope, while providing access to shared model properties, scopes can be nested to isolate application components
- Scope provides context against which expressions are evaluated
21. Explain what is DI (Dependency Injection ) and how an object or function can get a hold of its dependencies ?
DI or Dependency Injection is a software design pattern that deals with how code gets hold of its dependencies. In order to retrieve elements of the application which is required to be configured when module gets loaded , the operation “config” uses dependency injection.
These are the ways that object uses to hold of its dependencies
- Typically using the new operator, dependency can be created
- By referring to a global variable, dependency can be looked up
- Dependency can be passed into where it is required
22. Mention what are the advantages of using Angular.js framework ?
Advantages of using Angular.js as framework are
- Supports two way data-binding
- Supports MVC pattern
- Support static template and angular template
- Can add custom directive
- Supports REST full services
- Supports form validations
- Support both client and server communication
- Support dependency injection
- Applying Animations
- Event Handlers
23.Explain the concept of scope hierarchy? How many scope can an application have?
Each angular application consist of one root scope but may have several child scopes. As child controllers and some directives create new child scopes, application can have multiple scopes. When new scopes are formed or created they are added as a children of their parent scope. Similar to DOM, they also creates a hierarchical structure.
24.Explain what is the difference between AngularJS and backbone.js?
AngularJS combines the functionalities of most of the 3rd party libraries, it supports individual functionalities required to develop HTML5 Apps. While Backbone.js do their jobs individually.
25. What Is Data Binding? How Many Types Of Data Binding Directives Are Provided By AngularJS?
Data binding is the connection bridge between view and business logic (view model) of the application. Data binding in AngularJs is the automatic synchronization between the model and view. When the model changes, the view is automatically updated and vice versa. AngularJs support one-way binding as well as two-way binding.
AngularJS provides the following data binding directives:
1) <ng-bind>- It updates the text content of the specified HTML element with the value of the given expression. This text content gets updated when there is any change in the expression. It is very similar to double curly markup ( ) but less verbose.
It has the following Syntax.
<ANY ELEMENT ng-bind=”expression”> </ANY ELEMENT> |
2) <ng-bind-html>- It evaluates the expression and inserts the HTML content into the element in a secure way. To use this functionality, it has to use $sanitize service. For this, it is mandatory that $sanitize is available.
It has the following Syntax.
<ANY ELEMENT ng-bind-html=” expression “> </ANY ELEMENT> |
3) <ng-bind-template>- It replaces the element text content with the interpolation of the template. It can contain multiple double curly markups.
It has the following Syntax.
<ANY ELEMENT ng-bind-template=” … “> </ANY ELEMENT> |
4) <ng-non-bindable>- This directive informs AngularJS, not to compile or bind the contents of the current DOM element. It is useful in the case when the user wants to display the expression only and do not want to execute it.
It has the following Syntax.
<ANY ELEMENT ng-non-bindable > </ANY ELEMENT> |
5) <ng-model>- This directive can be bound with input, select, text area or any custom form control. It provides two-way data binding. It also provides validation behavior. It also retains the state of the control (like valid/invalid, touched/untouched and so on).
It has the following Syntax.
<input ng-bind=”expression”/> |
26. What Directives Are Used To Show And Hide HTML Elements In AngularJS?
Ans:
The directives used to show and hide HTML elements in the AngularJS are <ng-show> and <ng-hide>. They do this based on the result of an expression.
Its syntax is as follows.
<element ng-show=”expression”></element> |
When the expression for <ng-show> evaluates to true, then HTML element(s) are shown on the page, otherwise the HTML element is hidden. Similarly, <ng-hide> directive hides the HTML element if the expression evaluates to true.
Let’s take the following example.
<div ng-controller=”MyCtrl”>
<div ng-show=”data.isShow”>ng-show Visible</div> <div ng-hide=”data.isHide”>ng-hide Invisible</div> </div> <script> var app = angular.module(“app”, []); app.controller(“MyCtrl”, function ($scope) { $scope.data = {}; $scope.data.isShow = true; $scope.data.isHide = true; }); </script> |
27. Explain The Directives Ng-If, Ng-Switch, And Ng-Repeat?
A) <Ng-If>.
This directive can add/remove HTML elements from the DOM based on an expression. If the expression is true, it adds a copy of HTML elements to the DOM. If the expression evaluates to false, this directive removes the HTML element from the DOM.
<div ng-controller=”MyCtrl”>
<div ng-if=”data.isVisible”>ng-if Visible</div> </div> <script> var app = angular.module(“app”, []); app.controller(“MyCtrl”, function ($scope) { $scope.data = {}; $scope.data.isVisible = true; }); </script> |
B) <Ng-Switch>.
This directive can add/remove HTML elements from the DOM conditionally based on scope expression.
Child elements with the <ng-switch-when> directive will be displayed if it gets a match, else the element and its children get removed. It also allows defining a default section, by using the <ng-switch-default> directive. It displays a section if none of the other sections match.
Let’s see the following example that displays the syntax for <ng-switch>.
<div ng-controller=”MyCtrl”>
<div ng-switch on=”data.case”> <div ng-switch-when=”1″>Shown when case is 1</div> <div ng-switch-when=”2″>Shown when case is 2</div> <div ng-switch-default>Shown when case is anything else than 1 and 2</div> </div> </div> <script> var app = angular.module(“app”, []); app.controller(“MyCtrl”, function ($scope) { $scope.data = {}; $scope.data.case = true; }); </script> |
C) <Ng-Repeat>.
This directive is used to iterate over a collection of items and generate HTML from it.
<div ng-controller=”MyCtrl”>
<ul> <li ng-repeat=”name in names”> Angular JS Interview Questions and Answers </li> </ul> </div> <script> var app = angular.module(“app”, []); app.controller(“MyCtrl”, function ($scope) { $scope.names = [ ‘Mahesh’, ‘Raj’, ‘Diksha’ ]; }); </script> |
28. Explain The Set Of “Special” Variables Supported With <Ng-Repeat> Directive?
Answer.
The <ng-repeat> directive has a set of special variables that are useful while iterating the collection.
These variables are as follows.
- $index
- $first
- $middle
- $last
The “$index” contains the index of the element being iterated. The variables $first, $middle and $last returns a boolean value depending on whether the current item is the first, middle or last element in the collection being iterated.
<html>
<script src=”http://ajax.googleapis.com/ajax/libs/angularjs/1.4.8/angular.min.js”></script> <head> <script> var app = angular.module(“app”, []); app.controller(“ctrl”, function ($scope) { $scope.employees = [ { name: ‘A’, gender: ‘alphabet’ }, { name: ‘B’, gender: ‘number’ }, { name: ‘C’, gender: ‘alphanumeric’ }, { name: ‘D’, gender: ‘special character’ }]; }); </script> </head> <body ng-app=”app”> <div ng-controller=”ctrl”> <ul> <li ng-repeat=”employee in employees”> <div> is a . <span ng-if=”$first”> <strong>(first element found)</strong> </span> <span ng-if=”$middle”> <strong>(middle element found)</strong> </span> <span ng-if=”$last”> <strong>(last element found)</strong> </span> </div> </li> </ul> </div> </body> </html> |
The output is as follows.
1
2 3 4 |
A is a alphabet. (first element found)
B is a number. (middle element found) C is a alphanumeric. (middle element found) D is a special character. (last element found) |
29. What Is A Factory Method In AngularJS?
Ans:
A factory is a simple function which allows you to add some logic before creating the object. In the end, it returns the created object.
Syntax.
1 | app.factory(‘serviceName’,function(){ return serviceObj;}) |
Creating Service Using The Factory Method.
<script>
//creating module var app = angular.module(‘app’, []); //define a factory using factory() function app.factory(‘MyFactory’, function () { var serviceObj = {}; serviceObj.function1 = function () { //TO DO: }; serviceObj.function2 = function () { //TO DO: }; return serviceObj; }); </script> |
30.Explain What Is String Interpolation In AngularJS?
Ans:
During the compilation process, AngularJS compiler matches text and attributes using interpolate service to see if it contains embedded expressions.
During normal, digest life cycle, these expressions are updated and registered as watches.
31. Explain AngularJS Application Life-Cycle?
Understanding the life cycle of an AngularJS application makes it easier to learn about the way to design and implement the code. Apps life cycle consists of following three phases- bootstrap, compilation, and runtime.
These three phases of the life cycle occur each time a web page of an AngularJS application gets loaded in the browser. Let’s learn about each of the three phases in detail:
- The Bootstrap Phase –In this phase, the browser downloads the AngularJS javascript library. After this, AngularJS initializes its necessary components and the modules to which the ng-app directive points. Now that the module has loaded, required dependencies are injected into it and become available to the code within that module.
- The Compilation Phase –The second phase of the AngularJS life cycle is the HTML compilation stage. Initially, when a web page loads in the browser, a static form of the DOM gets loaded. During the compilation phase, this static DOM gets replaced with a dynamic DOM which represents the app view. There are two main steps – first, is traversing the static DOM and collecting all the directives. These directives are now linked to the appropriate JavaScript functionality which lies either in the AngularJS built-in library or custom directive code. The combination of directives and the scope, produce the dynamic or live view.
- The Runtime Data Binding Phase –This is the final phase of the AngularJS application. It survives until the user reloads or navigates away from the webpage. At this point, any changes in the scope get reflected in the view, and any changes in the view are directly updated in the scope, making the scope the single source of data for the view.
This shows that AngularJS behaves differently from traditional methods of binding data. The traditional methods combine a template with data, received from the engine and then manipulate the DOM each time there is any change in the data.
However, AngularJS compiles the DOM only once and then links the compiled template as necessary, making it much more efficient than the traditional methods.
32. Explain AngularJS Scope Life-Cycle?
After the angular app gets loaded into the browser, scope data passes through different stages called as its life cycle. Learning about this cycle helps us to understand the interaction between scope and other AngularJS components.
The scope data traverses through the following phases.
- Creation –This phase initializes the scope. During the bootstrap process, the $injector creates the root scope for the application. And during template linking, some directives create new child scopes. A digest loop also gets created in this phase that interacts with the browser event loop. This loop is responsible for updating DOM elements with the changes made to the model as well as executing any registered watcher functions.
- Watcher registration –This phase registers watchers for scope created in the above point. These watches propagate the model changes to the DOM elements, automatically. We can also register our own watcher’s on a scope by using the $watch() function.
- Model mutation –This phase occurs when there is any change in the scope data. When we do any modification in the angular app code, the scope function <$apply()> updates the model and then calls the <$digest()> function to update the DOM elements and the registered watches. However, when we change the scope inside the angular code like within controllers or services, angular internally calls <$apply()> function for us. But, when we do the changes to the scope outside the angular code, we have to call the <$apply()> function explicitly on the scope, to force the model and DOM to be updated correctly.
- Mutation observation –This phase occurs, when the digest loop execute the $digest() function at the end of $apply() call. When the $digest() function executes, it evaluates all watches for model changes. If there is a change in the value, $digest() calls the $watch listener and updates the DOM elements.
- Scope destruction –This phase occurs when the child scopes that are no longer needed, are removed from the browser’s memory by using the $destroy() function. It is the responsibility of the child scope creator to destroy them via scope.$destroy() API. This stops propagation of $digest calls into the child scopes and enables the browsers’ garbage collector to reclaim the unused memory.
33. What Is An Auto Bootstrap Process In AngularJS?
Ans:
AngularJS initializes automatically upon the “DOMContentLoaded” event or when the browser downloads the angular.js script and at the same time document.readyState is set to ‘complete’. At this point, AngularJS looks for the ng-app directive which is the root of Angular app compilation process.
If the ng-app directive is located, then AngularJS will do the following.
- Load the module associated with the directive.
- Create the application injector.
- Compile the DOM starting from the ng-app root element.
This process is auto-bootstrapping.
Following is the sample code that helps to understand it more clearly:
<html>
<body ng-app=”myApp”> <div ng-controller=”Ctrl”>Hello !</div> <script src=”https://ajax.googleapis.com/ajax/libs/angularjs/1.4.5/angular.min.js”></script> <script> var app = angular.module(‘myApp’, []); app.controller(‘Ctrl’, function($scope) { $scope.msg = ‘Welcome’; }); </script> </body> </html> |
34. What Is The Manual Bootstrap Process In AngularJS?
Ans:
Sometimes we may need to manually initialize Angular app in order to have more control over the initialization process. We can do that by using angular.bootstrap() function within angular.element(document).ready() function. AngularJS fires this function when the DOM is ready for manipulation.
The angular.bootstrap() function takes two parameters, the document, and module name injector.
Following is the sample code that helps to understand the concept more clearly.
<html>
<body> <div ng-controller=”Ctrl”>Hello !</div> <script src=”https://ajax.googleapis.com/ajax/libs/angularjs/1.4.5/angular.min.js”></script> <script> var app = angular.module(‘myApp’, []); app.controller(‘Ctrl’, function($scope) { $scope.msg = ‘Welcome’; }); //manual bootstrap process angular.element(document).ready(function () { angular.bootstrap(document, [‘myApp’]); }); </script> </body> </html> |
35. How To Bootstrap Your Angular App For Multiple Modules?
Ans:
Bootstrap for multiple modules can be achieved by using following two methods.
- Automatic bootstrap –AngularJS is automatically initialized for one module. When we have multiple modules, we combine them into a single module and thus the angular app will be automatically initialized for the newly created module. Other modules act as dependent modules for this newly created module.
Let’s take an example, suppose we have two modules: module1 and model2. To initialize the app automatically, based on these two modules following code is used:
<html>
<head> <title>Multiple modules bootstrap</title> <script src=”lib/angular.js”></script> <script> //module1 var app1 = angular.module(“module1”, []); app1.controller(“Controller1”, function ($scope) { $scope.name = “Welcome”; });
//module2 var app2 = angular.module(“module2”, []); app2.controller(“Controller2”, function ($scope) { $scope.name = “World”; });
//module3 dependent on module1 & module2 angular.module(“app”, [“module1”, “module2″]); </script> </head> <body> <!–angularjs autobootstap process–> <div ng-app=”app”> <h1>Multiple modules bootstrap</h1> <div ng-controller=”Controller2″> </div> <div ng-controller=”Controller1″> </div> </div> </body> </html> |
- Manual bootstrap –We can manually bootstrap the app by using angular.bootstrap() function, for multiple modules.
The above example can be rewritten for a manual bootstrap process as given below.
<html>
<head> <title>Multiple modules bootstrap</title> <script src=”lib/angular.js”></script> <script> //module1 var app1 = angular.module(“module1”, []); app1.controller(“Controller1”, function ($scope) { $scope.name = “Welcome”; });
//module2 var app2 = angular.module(“module2”, []); app2.controller(“Controller2”, function ($scope) { $scope.name = “World”; });
//manual bootstrap process angular.element(document).ready(function () { var div1 = document.getElementById(‘div1’); var div2 = document.getElementById(‘div2’);
//bootstrap div1 for module1 and module2 angular.bootstrap(div1, [‘module1’, ‘module2’]);
//bootstrap div2 only for module1 angular.bootstrap(div2, [‘module1’]); }); |
36. What Are Compile, Pre, And Post Linking In AngularJS?
Ans:
- A) Compile –It collects an HTML string or DOM into a template and produces a template function. It can then be used to link the scope and the template together.
AngularJS uses the compile function to change the original DOM before creating its instance and before the creation of scope.
Before discussing the Pre-Link and the Post-Link functions let’s see the Link function in detail.
- B) Link –It has the duty of linking the model to the available templates. AngularJS does the data binding to the compiled templates using Link.
Following is the Link syntax.
link: function LinkFn(scope, element, attr, ctrl){} |
where each of the four parameters is as follows.
- scope –It is the scope of the directive.
- element –It is the DOM element where the directive has to be applied.
- attr-It is the Collection of attributes of the DOM element.
- ctrl – It is the array of controllers required by the directive.
AngularJS allows setting the link property to an object also. The advantage of having an object is that we can split the link function into two separate methods called, pre-link and post-link.
- C) Post-Link –Execution of Post-Link function starts after the linking of child elements. It is safer to do DOM transformation during its execution. The post-link function is suitable to execute the logic.
- D) Pre-Link –It gets executed before the child elements are linked. It is not safe to do DOM transformation. As the compiler linking function will fail to locate the correct elements.
It is good to use the pre-link function to implement the logic that runs when AngularJS has already compiled the child elements. Also, before any of the child element’s post-link functions have been called.
Let’s see an example that talks about Compile, Pre-Link, and Post-Link functions.
<html>
<head> <title>Compile vs Link</title> <script src=”lib/angular.js”></script> <script type=”text/javascript”> var app = angular.module(‘app’, []); function createDirective(name) { return function(){ return { restrict: ‘E’, compile: function(tElem, tAttrs){ console.log(name + ‘: compile’); return { pre: function(scope, iElem, iAttrs){ console.log(name + ‘: pre link’); }, post: function(scope, iElem, iAttrs){ console.log(name + ‘: post link’); } } } } } }
app.directive(‘levelOne’, createDirective(‘levelOne’)); app.directive(‘levelTwo’, createDirective(‘levelTwo’)); app.directive(‘levelThree’, createDirective(‘levelThree’)); </script> </head> <body ng-app=”app”> <level-one> <level-two> <level-three> Hello Angular JS Interview Questions and Answers </level-three> </level-two> </level-one> </body> </html> |
||
Output:
Hello |
||
37. What Is A Controller In AngularJS?
Ans:
A Controller is a set of JavaScript functions which is bound to a specified scope, the ng-controller directive. Angular creates a new instance of the Controller object to inject the new scope as a dependency. The role of the Controller is to expose data to our view via $scope and add functions to it, which contains business logic to enhance view behavior.
Controller Rules.
- A Controller helps in setting up the initial state of the scope object and define its behavior.
- The Controller should not be used to manipulate the DOM as it contains only business logic. Rather, for manipulating the DOM, we should use data binding and directives.
- Do not use Controllers to format input. Rather, using angular form controls is recommended for that.
- Controllers should not be used to share code or states. Instead, use angular services for it.
Steps For Creating A Controller.
- It needs ng-controller directive.
- Next step is to add Controller code to a module.
- Name your Controller based on functionality. Its name should follow camel case format (i.e. SampleController).
- Set up the initial state of the scope object.
Declaring a Controller using ng-Controller directive.
<div ng-app=”mainApp” ng-controller=”SampleController”>
</div> |
Following code displays the definition of SampleController.
<script>
function SampleController($scope) { $scope.sample = { firstSample: “INITIAL”, lastSample: “Initial”,
fullName: function() { var sampleObject; sampleObject = $scope.sample; return sampleObject.firstSample + ” ” + sampleObject.lastSample; } }; } </script> |
38. What Does A Service Mean In AngularJS? Explain Its Built-In Services?
Ans:
Services are functions that are bound to perform specific tasks in an application.
- It gives us a method, that helps in maintaining the angular app data for its lifetime.
- It gives us methods, that facilitate to transfer data across the controllers in a consistent way.
- It is a singleton object and its instance is created only once per application.
- It is used to organize and share, data and function across the application.
Two main execution characteristics of angular services are that they are Singleton and lazy instantiated.
- Lazily instantiated –
It means that AngularJS instantiates a service only when a component of an application needs it. This is done by using dependency injection method, that makes the Angular codes, robust and less error prone.
- Singletons –
Each application component dependent on the service, work with the single instance of the service created by the AngularJS.
Let us take an example of a very simple service that calculates the square of a given number:
var CalculationService = angular.module(‘CalculationService’, [])
.service(‘Calculation’, function () { this.square = function (a) { return a*a}; }); |
AngularJS Internal Services –
AngularJS provides many built-in services. Each of them is responsible for a specific task. Built-in services are always prefixed with the $ symbol.
Some of the commonly used services in any AngularJS application are as follows:
- $http –used to make an Ajax call to get the server data.
- $window –Provides a reference to a DOM object.
- $Location –Provides reference to the browser location.
- $timeout –Provides a reference to window.set timeout function.
- $Log –used for logging.
- $sanitize –Used to avoid script injections and display raw HTML in the page.
- $Rootscope –Used for scope hierarchy manipulation.
- $Route –Used to display browser based path in browser URL.
- $Filter –Used for providing filter access.
- $resource –Used to work with Restful API.
- $document –Used to access the window. Document object.
- $exceptionHandler –Used for handling exceptions.
- $q –Provides a promise object.
- $cookies –This service is useful to write, read and delete browser cookies.
- $parse –This service is useful to convert AngularJS expression into a function.
- $cacheFactory –This service evaluates the specified expression when the user changes the input.
39. What Are Different Ways To Create Service In AngularJS?
There are 5 different ways to create services in AngularJS.
- Value
- Factory
- Service
- Provider
- Constant
Let’s discuss, each of the above AngularJS service types one by one with code example:
1. AngularJS Value.
It is the simplest service type supported by AngularJS that we can create and use. It is similar to a key-value pair or like a variable having a value. It can store only a single value. Let’s take an example and create a service that displays username:
var app=angular.module(“app”,[]);
app.value(“username”,”Madhav”); |
Code to use “Value”:
We can use this service anywhere by using dependency injection. Following example injects the service in a controller:
app.controller(“MainController”,function($scope, username){
$scope.username=username; }); |
In the above example, we have created a Value service “username” and used it in MainController.
2. AngularJS Factory.
Value service may be very easy to write but, it lacks many important features. So, the next service type we will look at is “Factory” service. After its creation, we can even inject other services into it. Unlike Value service, we cannot add any dependency in it.
Let’s take an example to create a Factory service.
app.factory(“username”,function(){
var name=”John”; return { name:name } }); |
The above code shows that Factory service takes “function” as an argument. We can inject any number of dependencies or methods in this “function” as required by this service. This function must return some object. In our example, it returns an object with the property name. Now, let us look, as to how we can use this service:
Code to use “Factory”:
The function returns an object from service which has a property name so we can access it and use it anywhere. Let’s see how we can use it in the controller:
app.controller(“MainController”,function($scope, username){
$scope.username=username.name; }); |
We are assigning the username from factory service to our scope username.
3. AngularJS Service.
It works same as the “Factory” service. But, instead of a function, it receives a Javascript class or a constructor function as an argument. Let’s take an example. Suppose we have a function:
1
2 3 |
function MyExample(num){
this.variable=”value”; } |
Now, we want to convert the function into a service. Let’s take a look at how we can do this with “Factory” method:
app.factory(“MyExampleService”,[“num” ,function(num){
return new MyExample(num); }]); |
Thus in this way, we will create its new instance and return it. Also, we have injected <num> as a dependency in Factory service. Now, let’s see how we can do this using Service type:
1 | app.service(“MyExampleService”,[“num”, MyExample]); |
Thus, we have called the service method on the module and provided its name, dependency, and the name of the function in an array.
4. AngularJS Provider.
It is the parent of all the service types supported by AngularJS, except the “Constant” that we will discuss in the next section. It is the core of all the service types. Thus we can say that other services work on top of it. It allows us to create a configurable service that must implement the <$get> method.
We use this service to expose the API that is responsible for doing the application-wide configuration. The configuration should complete before starting the application.
Let’s take an example.
app.provider(‘authentication’, function() {
var username = “John”; return { set: function(newUserName) { username = newUserName; }, $get: function() { function getUserName() { return username; } return { getUserName: getUserName }; } }; }); |
This example initializes a provider with its name as “authentication”. It also implements a <$get> function, which returns a method “getUsername” which in turn returns the private variable called username. This also has a setter, using it we can set the username on application startup as follows:
app.config([“authenticationProvider”, function(authenticationProvider) {
authenticationProvider.set(“Mihir”); }]); |
5. AngularJS Constant.
As the name suggests, this service helps us to declare constants in our application. We can then use them wherever needed, just by adding it as a dependency. There are many places, where we use constants like some base URLs, application name, etc.
We just define them once and use them anywhere as per our need. Thus, this technique allows us to write the definition at one place. If there is any change in the value later, we have to do the modifications at one place only.
Here is an example of how we can create constants:
app.constant(‘applicationName’, ‘Service Tutorials’); |
40. What Is The Difference Between The $Watch, $Digest, And $Apply?
Ans:
In AngularJS $scope object is having different functions like $watch(), $digest() and $apply() and we will call these functions as central functions. The AngularJS central functions $watch(), $digest(), and $apply() are used to bind data to variables in view and observe changes happening in variables.
A) $Watch() –
The use of this function is to observe changes in a variable on the $scope. It triggers a function call when the value of that variable changes. It accepts three parameters: expression, listener, and equality object. Here, listener and equality objects are optional parameters.
1 | $watch(watchExpression, listener, [objectEquality]). |
Following is the example of using $watch() function in AngularJS applications.
<html>
<head> <title>AngularJS Watch</title> <script src=”lib/angular.js”></script> <script> var myapp = angular.module(“myapp”, []); var myController = myapp.controller(“myController”, function ($scope) { $scope.name = ‘dotnet-tricks.com’; $scope.counter = 0; //watching change in name value $scope.$watch(‘name’, function (newValue, oldValue) { $scope.counter = $scope.counter + 1; }); }); </script> </head> <body ng-app=”myapp” ng-controller=”myController”> <input type=”text” ng-model=”name” /> <br /><br /> Counter: </body> </html> |
B) $Digest() –
This function iterates through all the watch list items in the $scope object, and its child objects (if it has any). When $digest() iterates over the watches, it checks if the value of the expression has changed or not. If the value has changed, AngularJS calls the listener with the new value and the old value.
The $digest() function is called whenever AngularJS thinks it is necessary. For example, after a button click, or after an AJAX call. You may have some cases where AngularJS does not call the $digest() function for you. In that case, you have to call it yourself.
Following is the example of using $digest() function in AngularJS applications:
<html>
<head> <title>AngularJS Digest Example</title> <script src=”lib/jquery-1.11.1.js”></script> <script src=”lib/angular.js”></script> </head> <body ng-app=”app”> <div ng-controller=”Ctrl”> <button class=”digest”>Digest my scope!</button> <br /> <h2>obj value : </h2> </div> <script> var app = angular.module(‘app’, []); app.controller(‘Ctrl’, function ($scope) { $scope.obj = { value: 1 }; $(‘.digest’).click(function () { console.log(“digest clicked!”); console.log($scope.obj.value++); //update value $scope.$digest(); }); }); </script> </body> </html> |
C) $Apply() –
AngularJS automatically updates the model changes which are inside AngularJS context. When you apply changes to any model, that lies outside of the Angular context (like browser DOM events, setTimeout, XHR or third party libraries), then you need to inform the Angular about the changes by calling $apply() manually. When the $apply() function call finishes, AngularJS calls $digest() internally, to update all data bindings.
Following are the key differences between $apply() and $digest().
- Its use is to update the model properties forcibly.
- The $digest() method evaluates the watchers for the current scope. However, the $apply() method is used to evaluate watchers for root scope, that means it’s for all scopes.
Following is the example of using the $apply() function in AngularJS applications.
<html>
<head> <title>AngularJS Apply Example</title> <script src=”lib/angular.js”></script> <script> var myapp = angular.module(“myapp”, []); var myController = myapp.controller(“myController”, function ($scope) { $scope.datetime = new Date(); $scope.updateTime = function () { $scope.datetime = new Date(); } //outside angular context document.getElementById(“updateTimeButton”).addEventListener(‘click’, function () { //update the value $scope.$apply(function () { console.log(“update time clicked”); $scope.datetime = new Date(); console.log($scope.datetime); }); }); }); </script> </head> <body ng-app=”myapp” ng-controller=”myController”> <button ng-click=”updateTime()”>Update time – ng-click</button> <button id=”updateTimeButton”>Update time</button> <br />
</body> </html> |
[/vc_column_text][vc_single_image image=”1811″ img_size=”full” onclick=”custom_link” img_link_target=”_blank” link=”http://www.mytectra.com/aws-training-in-bangalore.html”][/vc_column][/vc_row][vc_row][vc_column][vc_column_text]
41. Which One Handles Exception Automatically Between $Digest And $Apply?
Ans:
When an error occurs in one of the watchers, $digest() cannot handle them via $exceptionHandler service. In that case, you have to handle the exception yourself. However, $apply() uses try catch block internally to handle errors. But, if an error occurs in one of the watchers, then it transfers the errors to $exceptionHandler service.
Code for $apply() function.
$apply(expr) {
try { return $eval(expr); } catch (e) { $exceptionHandler(e); } finally { $root.$digest(); } } |
42. Explain $Watch(), $Watchgroup() And $WatchCollection() Functions Of Scope?
A) $Watch.
Its use is to observe the changes in the variable on the $scope. It accepts three arguments – expression, listener, and equality object. The listener and Equality object are optional parameters.
$watch(watchExpression, listener, [objectEquality]) |
Here, <watchExpression> is the expression to be observed in the scope. This expression gets called on every $digest() and returns a value for the listener to monitor.
The listener defines a function which gets called when watchExpression changes its value. In case, its value does not change then the listener will not be called. The objectEquality is a boolean type to compare the objects for equality using angular.equals.
scope.name = ‘shailendra’;
scope.counter = 0; scope.$watch(‘name’, function (newVal, oldVal) { scope.counter = scope.counter + 1; }); |
B) $Watchgroup.
This function was introduced in Angular1.3. It works in the same way as $watch() function except that the first parameter is an array of expressions.
1 | $watchGroup(watchExpression, listener) |
The listener is also an array containing the new and old values of the variables that are being watched. The listener gets called whenever any expression contained in the watchExpressions array changes.
$scope.teamScore = 0;
$scope.time = 0; $scope.$watchGroup([‘teamScore’, ‘time’], function(newVal, oldVal) { if(newVal[0] > 20){ $scope.matchStatus = ‘win’; } else if (newVal[1] > 60){ $scope.matchStatus = ‘times up’; }); |
C) $WatchCollection.
This use of this function is to watch the properties of an object. It gets fired when there is any change in the properties. It takes an object as the first parameter and watches the properties of the object.
Javascript
$watchCollection(obj, listener) |
The listener is called whenever there is any change in the obj.
Javascript
$scope.names = [‘shailendra’, ‘deepak’, ‘mohit’, ‘kapil’];
$scope.dataCount = 4; $scope.$watchCollection(‘names’, function (newVal, oldVal) { $scope.dataCount = newVal.length; }); |
43. What Are The Form Validations Supported By AngularJS?
AngularJS allows form validation on the client-side in a simplistic way. First of all, it monitors the state of the form and its input fields. Secondly, it observes any change in the values and notifies the same to the user.
Let’s discuss the different input field validations along with examples.
A) Required Field Validation.
By using “Required Field” validation we can prevent, form submission with a null value. It’s mandatory for the user to fill the form fields.
The syntax for required field validation is as follows.
Javascript
<input type=”text” required /> |
Example Code.
Javascript
<form name=”myForm”>
<input name=”myInput” ng-model=”myInput” required> </form> <p>The input’s valid state is:</p> <h1></h1> |
B) Minimum & Maximum Field Length Validations.
To prevent the user from providing less or excess number of characters in the input field, we use Minimum & Maximum length validation. The AngularJS directive used for Minimum & Maximum length validations are <ng-minlength> and <ng-maxlength>. Both of these attributes take integer values. The <ng-minlength> attribute is used to set the number of characters a user is limited to, whereas the <ng-maxlength> attribute sets the maximum numbers of characters that a user is allowed to enter.
It has the following syntax.
Javascript
<input type=”text” ng-minlength=5 />
<input type=”text” ng-maxlength=10 /> |
Example code:
Javascript
<label>User Message:</label>
<textarea type=”text” name=”userMessage” ng-model=”message” ng-minlength=”100″ ng-maxlength=”1000″ required> </textarea> <div ng-messages=”exampleForm.userMessage.$error”> <div ng-message=”required”>This field is required</div> <div ng-message=”minlength”>Message must be over 100 characters</div> <div ng-message=”maxlength”>Message must not exceed 1000 characters</div> </div> |
C) Matches Pattern Validation.
AngularJS provides a <ng-pattern> directive to ensure that input fields match the regular expressions that are passed into the attribute.
It has the following syntax.
Javascript
<input type=”text” ng-pattern=”[a-zA-Z]” /> |
To activate the error message in <ng-pattern> we pass the value of pattern into ng-message.
Example code.
Javascript
<label>Phone Number:</label>
<input type=”email” name=”userPhoneNumber” ng-model=”phoneNumber” ng-pattern=”/^[\+]?[(]?[0-9]{3}[)]?[-\s\.]?[0-9]{3}[-\s\.]?[0-9]{4,6}$/” required/> <div ng-messages=”exampleForm.userPhoneNumber.$error”> <div ng-message=”required”>This field is required</div> <div ng-message=”pattern”>Must be a valid 10 digit phone number</div> </div> |
D) Email Validation.
In order to validate an email id, AngularJS provides ng-model directive. Using the following syntax we can validate an email id from any input field.
Javascript
<input type=”email” name=”email” ng-model=”user.email” /> |
Example code.
Javascript
<label>Email Address:</label>
<input type=”email” name=”userEmail” ng-model=”email” required /> <div ng-messages=”exampleForm.userEmail.$error”> <div ng-message=”required”>This field is required</div> <div ng-message=”email”>Your email address is invalid</div> </div> |
E) Number Validation.
To validate an input against Number we can use ng-model directive from AngularJS.
Its syntax is as follows.
Javascript
<input type=”number” name=”personage” ng-model=”user.age” /> |
F) URL Validation.
To validate an input field for URL, we can use the following syntax.
<input type=”url” name=”weblink” ng-model=”user.facebook_url” /> |
Example Code.
Javascript
<div ng-app=”urlInputExample”>
<form name=”myForm” ng-controller=”UrlController”> <label for=”exampleInput”>Enter Email</label> <input type=”url” name=”input” ng-model=”example.url” required /> <p style=”font-family:Arial;color:red;background:steelblue;padding:3px;width:350px;” ng-if=’!myForm.input.$valid’>Enter Valid URL</p> </form> </div> |
44. How Do You Exchange Data Among Different Modules Of Your Angular JS Application?
Ans:
There are a no. of ways in Angular to share data among modules. A few of them are as follows.
- The most common method is to create an Angular service to hold the data and dispatch it across the modules.
- Angular has a matured event system which provides $broadcast(), $emit() and $on() methods to raise events and pass data among the controllers.
- We can also use $parent, $nextSibling, and $ controllerAs to directly access the controllers.
- Variables defined at the root scope level ($rootScope) are available to the controller scope via prototypical inheritance. But they behave like globals and hard to maintain.
45. How Would You Use An Angular Service To Pass Data Between Controllers? Explain With Examples?
Using a service is the best practice in Angular to share data between controllers. Here is a step by step example to demonstrate data transfer.
We can prepare the data service provider in the following manner.
Javascript
app.service(‘dataService’, function() {
var dataSet = [];
var addData = function(newData) { dataSet.push(newData); };
var getData = function(){ return dataSet; };
return { addData: addData, getData: getData }; }); |
Now, we’ll inject the service dependency into the controllers.
Say, we have two controllers – pushController and popController.
The first one will add data by using the data service provider’s addData method. And the latter will fetch this data using the service provider’s getData method.
Javascript
app.controller(‘pushController’, function($scope, dataService) {
$scope.callToAddToProductList = function(currObj){ dataService.addData(currObj); }; });
app.controller(‘popController’, function($scope, dataService) { $scope.dataSet = dataService.getData(); }); |
46. How Will You Send And Receive Data Using The Angular Event System? Use Methods Like $Broadcast And $On To Send Data Across?
We can call the $broadcast method using the $rootScope object and send any data we want.
Javascript
$scope.sendData = function() {
$rootScope.$broadcast(‘send-data-event’, data); } |
To receive data, we can use the $scope object inside a controller.
Javascript
$scope.$on(‘send-data-event’, function(event, data) {
// process the data. }); |
47. How Do You Switch To Different Views From A Controller Function?
By using the $location service from the Controller function, we can traverse across multiple views. Here is an example to explain.
In the below index.html file, we are calling the Controller functions to switch to different views.
XHTML
<div ng-controller=”viewController”>
<div ng-click=”showView(‘edit’)”> Edit </div> <div ng-click=”showView(‘search’)”> Search </div> </div> |
Below is the code from the viewController.js file implementing the showView method.
JavaScript
function viewController ($scope, $location) {
$scope.showView = function(view){ $location.path(view); // Show the view } } |
48. What Would You Do To Limit A Scope Variable To Have One-Time Binding?
Ans:
By prefixing the “::” operator to the scope variable. It’ll make sure the candidate is aware of the available variable bindings in AngularJS.
49. What Is The Difference Between One-Way Binding And Two-Way Binding?
The main difference between one-way binding and two-way binding is as follows.
- In one-way binding, the scope variable in the HTML gets initialized with the first value its model specifies.
- In two-way binding, the scope variable will change its value whenever the model gets a different value.
50. Which Angular Directive Would You Use To Hide An Element From The DOM Without Modifying Its Style?
It is the conditional ngIf Directive which we can apply to an element. Whenever the condition becomes false, the ngIf Directive removes it from the DOM.
51.When To Use Factory?
It is just a collection of functions, like a class. Hence, it can be instantiated in different controllers when you are using it with a constructor function.
You May Also Like
These Related Stories
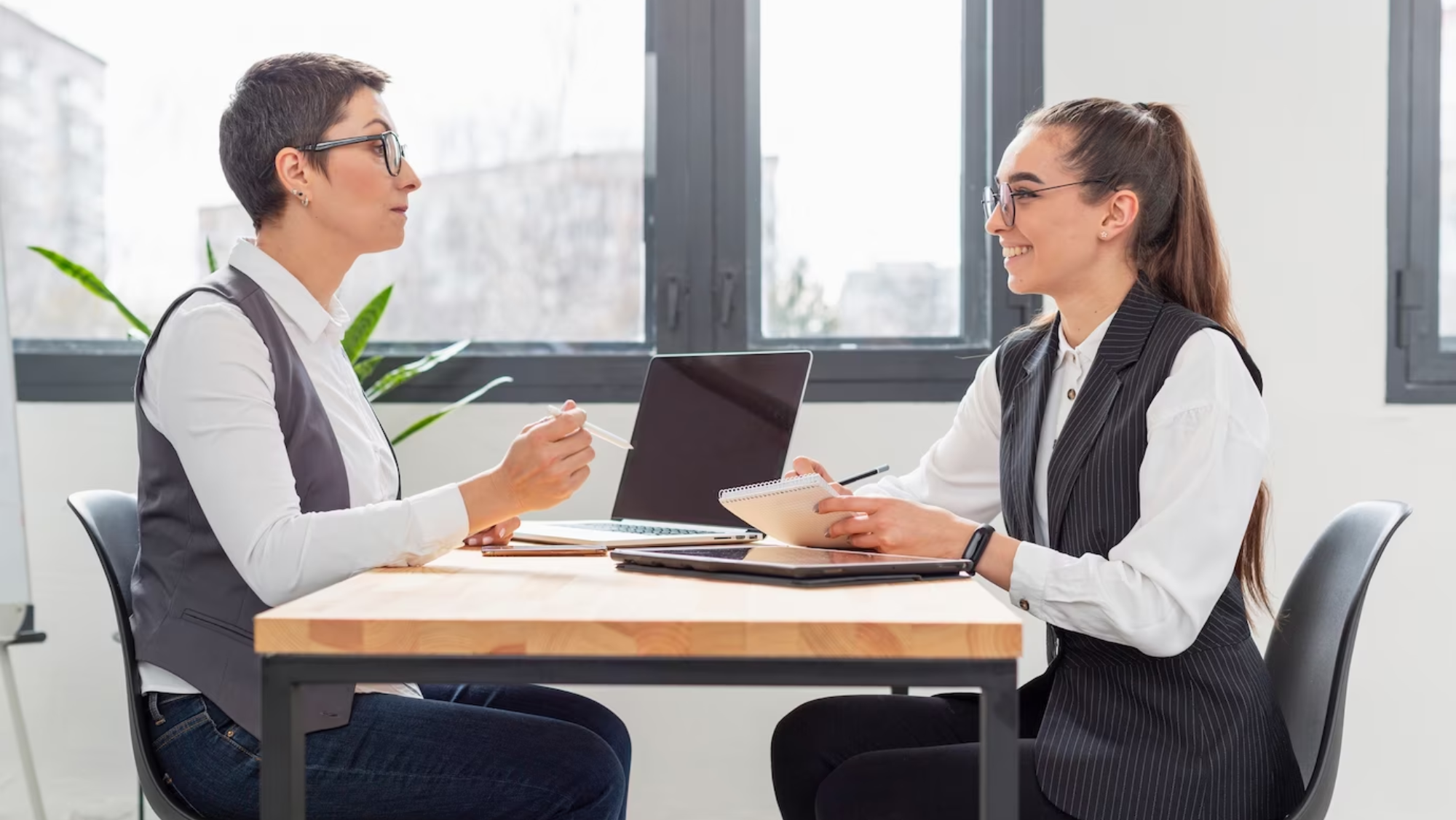
Top 25 Snowflake Interview Questions and Answers
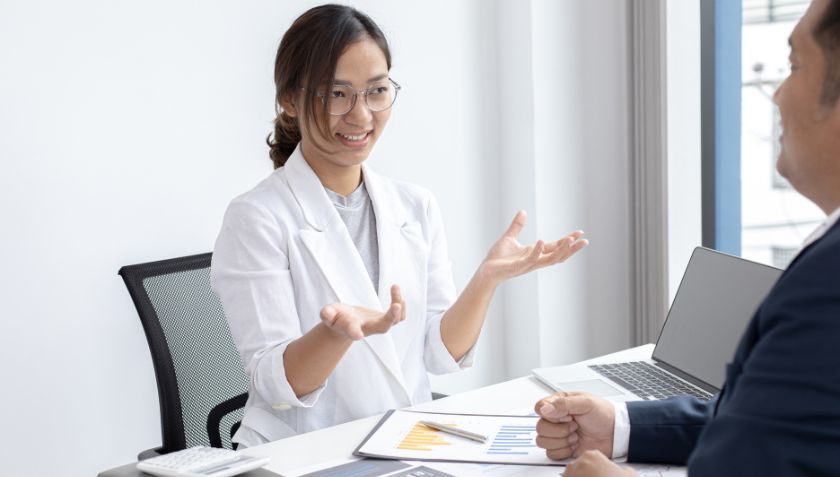
Ajax Interview Questions and Answers
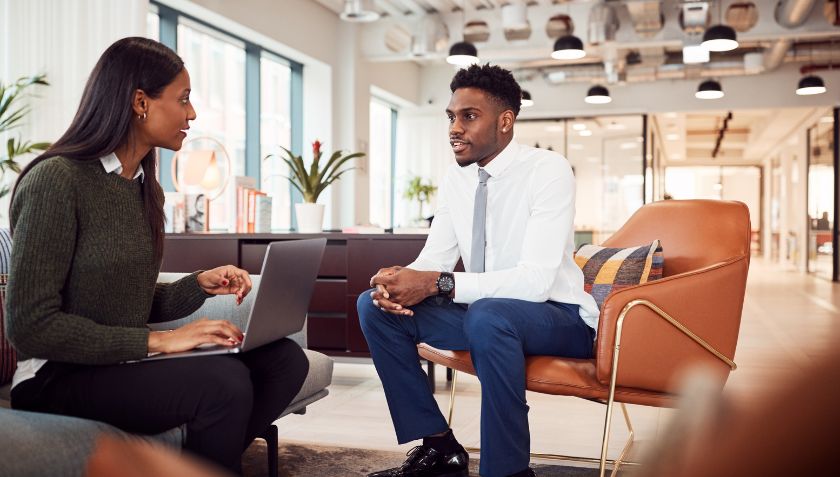
No Comments Yet
Let us know what you think